用c语言写一段代码 :1、初始化单链表,构造一个带头结点的单链表L; 2、建立长度为n的单链表并输出; 3、查找元素e,返回其序号; 4、在第i个位置插入新元素e; 5、删除第i个位置的元素,并由e返回其值。
时间: 2024-10-17 19:10:46 浏览: 23
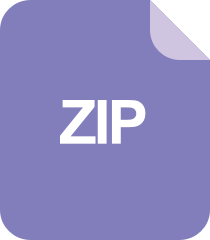
链表-使用C语言实现带头结点的单链表.zip
以下是使用C语言实现上述功能的一个简短示例:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表结构体
typedef struct Node {
int data; // 数据域
struct Node* next; // 指针域
} Node;
// 初始化带头结点的单链表
Node* createList() {
Node* head = (Node*)malloc(sizeof(Node));
if (!head) {
printf("Memory allocation failed.\n");
return NULL;
}
head->data = 0;
head->next = NULL;
return head;
}
// 插入节点
void insertNode(Node** head, int i, int e) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (!newNode) {
printf("Memory allocation failed.\n");
return;
}
newNode->data = e;
newNode->next = *head;
for (int j = 0; j < i - 1 && *head != NULL; j++) {
(*head) = (*head)->next;
}
(*head) = newNode;
}
// 查找元素序号
int searchElement(Node* head, int e) {
int index = 0;
while (head != NULL) {
if (head->data == e)
return index;
head = head->next;
index++;
}
return -1; // 如果未找到则返回-1
}
// 删除节点并获取值
int deleteNode(Node** head, int i) {
if (*head == NULL) return -1;
if (i == 0) { // 如果要删除的是头节点
Node* temp = *head;
*head = (*head)->next;
free(temp);
return temp->data;
}
Node* current = *head;
for (int j = 0; j < i - 1 && current != NULL; j++)
current = current->next;
if (current == NULL || current->next == NULL)
return -1; // 没有找到对应的位置
Node* temp = current->next;
current->next = temp->next;
free(temp);
return temp->data;
}
// 输出链表
void printList(Node* head) {
Node* temp = head;
while (temp != NULL) {
printf("%d -> ", temp->data);
temp = temp->next;
}
printf("NULL\n");
}
int main() {
int n, e, i;
printf("Enter the length of the list (n): ");
scanf("%d", &n);
Node* head = createList();
if (head) {
printf("Enter elements and their positions to insert or delete:\n");
for (int j = 0; j < n; j++) {
printf("At position %d, enter element: ", j);
scanf("%d", &e);
insertNode(&head, j+1, e); // 从1开始计数
}
printf("Initial list: ");
printList(head);
printf("Enter an element to find its position: ");
scanf("%d", &e);
int position = searchElement(head, e);
if (position != -1)
printf("Element found at position: %d\n", position + 1);
else
printf("Element not found.\n");
printf("Enter a position to delete an element: ");
scanf("%d", &i);
int deletedValue = deleteNode(&head, i);
if (deletedValue != -1)
printf("Deleted element was: %d\n", deletedValue);
else
printf("Deletion failed.\n");
}
return 0;
}
```
阅读全文
相关推荐
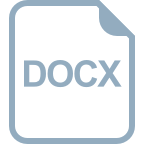
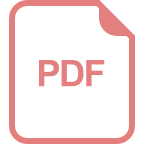















