用c语言1.定义循环队列。 2.分别定义队列的基本操作(初始化、判断空满、查询长度、入队、出队、遍历队列、取队头数据元素)。 3.设计一个测试主函数进行测试。 4.对程序的运行结果进行分析。
时间: 2024-10-28 18:09:29 浏览: 30
在C语言中,我们可以使用数组来实现一个简单的循环队列。首先,定义一个结构体表示队列元素,然后创建两个数组:一个存放数据,另一个作为索引指示当前队列的位置。
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct {
int data; // 存储元素的数据部分
} Node;
#define QUEUE_SIZE 10
Node queue[QUEUE_SIZE]; // 队列数组
int front = -1, rear = -1; // front表示队首,rear表示队尾
// 初始化队列
void initQueue() {
front = rear = -1;
}
// 判断队列是否为空
int isEmpty() {
return front == rear;
}
// 判断队列是否已满
int isFull() {
return (front + 1) % QUEUE_SIZE == rear; // 使用循环队列的概念,索引溢出后会回到0
}
// 查询队列长度
int getQueueLength() {
if (isEmpty()) {
return 0;
} else {
return rear - front + 1;
}
}
// 入队操作
void enqueue(int value) {
if (isFull()) {
printf("Queue is full.\n");
} else {
rear = (rear + 1) % QUEUE_SIZE;
queue[rear].data = value;
if (front == -1) {
front = rear;
}
printf("Enqueue: Added %d\n", value);
}
}
// 出队操作
int dequeue() {
if (isEmpty()) {
printf("Queue is empty.\n");
} else {
int temp = queue[front].data;
if (front == rear) { // 如果队列为单元素,则更新 front 和 rear
front = rear = -1;
} else {
front = (front + 1) % QUEUE_SIZE;
}
printf("Dequeue: Removed %d\n", temp);
return temp;
}
}
// 遍历队列
void displayQueue() {
if (isEmpty()) {
printf("Queue is empty.\n");
} else {
for (int i = front; i != rear; i = (i + 1) % QUEUE_SIZE) {
printf("Element at index %d: %d\n", i, queue[i].data);
}
}
}
// 主函数测试
int main() {
initQueue();
enqueue(1);
enqueue(2);
enqueue(3);
displayQueue();
printf("Queue length: %d\n", getQueueLength());
printf("%d removed from the queue.\n", dequeue());
displayQueue();
return 0;
}
```
运行这个程序,你会看到插入、删除和遍历队列的操作效果。如果尝试在队列满的情况下插入元素,它会提示“Queue is full”。同样,当队列为空时,某些操作(如出队和获取长度)会返回错误信息。
阅读全文
相关推荐
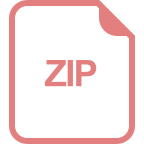
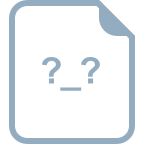
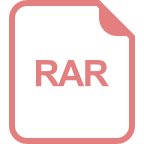















