用c语言写循环队列的基本操作实现(初始化,入队,出队,求队列长度,取队头元素,判断队空,遍历,清空,销毁等),要求建立菜单进行操作选择
时间: 2024-12-17 22:50:54 浏览: 14
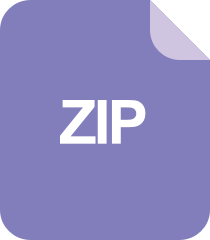
C语言实现使用静态数组实现循环队列源码.zip
在C语言中,我们可以使用数组和两个指针(一个指向当前队尾位置,另一个指向下一个待入队的位置)来实现循环队列。以下是一个简单的循环队列实现及其菜单驱动的操作:
```c
#include <stdio.h>
#define MAX_SIZE 50
typedef struct {
int data[MAX_SIZE];
int front, rear; // 前面的索引是front,后面的索引是rear+1
} circular_queue;
// 初始化队列
void init(circular_queue *cq) {
cq->front = cq->rear = -1;
}
// 入队操作
void enqueue(circular_queue *cq, int value) {
if (cq->rear == (MAX_SIZE - 1)) { // 队满
printf("Queue is full.\n");
} else {
if (cq->front == -1) {
cq->front = 0; // 空队列时,front置零
}
cq->data[cq->rear] = value;
cq->rear = (cq->rear + 1) % MAX_SIZE; // 循环队列的后指针更新
}
}
// 出队操作
int dequeue(circular_queue *cq) {
if (cq->empty()) {
printf("Queue is empty.\n");
return -1; // 返回-1表示队列为空
} else {
int value = cq->data[cq->front];
cq->front = (cq->front + 1) % MAX_SIZE; // 更新前指针
return value;
}
}
// 判断队空
bool empty(circular_queue *cq) {
return (cq->front == cq->rear);
}
// 获取队列长度
int length(circular_queue *cq) {
if (cq->front > cq->rear) {
return (MAX_SIZE - cq->front) + cq->rear; // 使用模运算处理环形
} else {
return (cq->rear - cq->front); // 非环形情况
}
}
// 显示队列元素
void display(circular_queue *cq) {
if (cq->empty()) {
printf("Queue is empty.\n");
} else {
for (int i = cq->front; i != cq->rear; i = (i + 1) % MAX_SIZE) {
printf("%d ", cq->data[i]);
}
}
}
// 清空队列
void clear(circular_queue *cq) {
cq->front = cq->rear = -1;
}
// 销毁队列
void destroy(circular_queue *cq) {
free(cq->data);
}
int main() {
circular_queue cq;
int choice, val;
while (1) {
printf("\nCircular Queue Menu:\n1. Initialize\n2. Enqueue\n3. Dequeue\n4. Check queue length\n5. Display elements\n6. Clear queue\n7. Destroy\n8. Exit\n");
scanf("%d", &choice);
switch (choice) {
case 1: init(&cq); break;
case 2: printf("Enter a value to enqueue: "); scanf("%d", &val); enqueue(&cq, val); break;
case 3: val = dequeue(&cq); break;
case 4: printf("Queue length: %d\n", length(&cq)); break;
case 5: display(&cq); break;
case 6: clear(&cq); break;
case 7: destroy(&cq); return 0; // 销毁队列并退出
case 8: return 0; // 退出程序
default: printf("Invalid choice. Try again.\n");
}
}
}
```
这个程序实现了循环队列的基本操作,并通过一个简单的命令行菜单让用户选择如何操作。请注意,在实际应用中,对于大型数据结构,可能需要更完善的错误处理和内存管理。
阅读全文
相关推荐
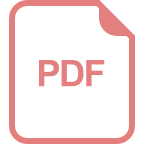
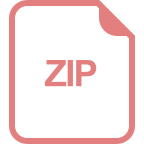

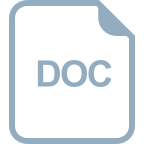













