RSA算法加解密python
时间: 2024-12-12 08:11:18 浏览: 2
RSA算法是一种非对称加密算法,广泛应用于数据加密和数字签名。RSA算法的安全性基于大数分解的困难性。以下是使用Python进行RSA加解密的示例代码:
首先,我们需要安装`cryptography`库,可以使用以下命令进行安装:
```bash
pip install cryptography
```
然后,我们可以使用以下代码进行RSA加解密:
```python
from cryptography.hazmat.primitives.asymmetric import rsa, padding
from cryptography.hazmat.primitives import serialization, hashes
# 生成RSA密钥对
def generate_keys():
private_key = rsa.generate_private_key(
public_exponent=65537,
key_size=2048,
)
public_key = private_key.public_key()
return private_key, public_key
# RSA加密
def encrypt(public_key, plaintext):
ciphertext = public_key.encrypt(
plaintext.encode('utf-8'),
padding.OAEP(
mgf=padding.MGF1(algorithm=hashes.SHA256()),
algorithm=hashes.SHA256(),
label=None
)
)
return ciphertext
# RSA解密
def decrypt(private_key, ciphertext):
plaintext = private_key.decrypt(
ciphertext,
padding.OAEP(
mgf=padding.MGF1(algorithm=hashes.SHA256()),
algorithm=hashes.SHA256(),
label=None
)
)
return plaintext.decode('utf-8')
# 示例使用
if __name__ == "__main__":
private_key, public_key = generate_keys()
message = "Hello, RSA!"
print(f"Original message: {message}")
encrypted_message = encrypt(public_key, message)
print(f"Encrypted message: {encrypted_message}")
decrypted_message = decrypt(private_key, encrypted_message)
print(f"Decrypted message: {decrypted_message}")
```
### 代码说明:
1. **生成密钥对**:使用`rsa.generate_private_key`生成私钥,并通过`private_key.public_key()`生成公钥。
2. **加密**:使用公钥进行加密,`padding.OAEP`提供了一种安全的填充方式。
3. **解密**:使用私钥进行解密,`padding.OAEP`用于验证填充。
###
阅读全文
相关推荐
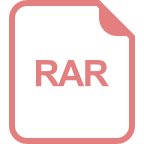
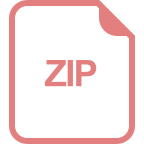
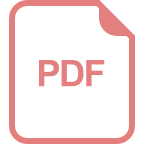


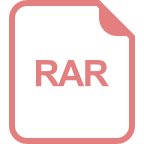
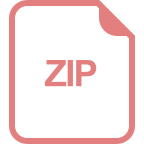











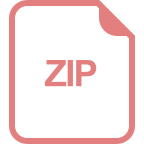