rsa加密解密算法实验简单python代码
时间: 2024-10-09 13:07:25 浏览: 80
RSA(Rivest-Shamir-Adleman)是一种非对称加密算法,它利用两个密钥(公钥和私钥)进行信息的加密和解密。以下是使用Python实现RSA加密和解密的一个简化版本:
```python
import math
from Crypto.Util.number import inverse
# RSA关键生成
def gcd(a, b):
while b != 0:
a, b = b, a % b
return a
def egcd(a, b):
if a == 0:
return (b, 0, 1)
else:
g, y, x = egcd(b % a, a)
return (g, x - (b // a) * y, y)
def modinv(a, m):
g, x, _ = egcd(a, m)
if g == 1:
return x % m
def generate_keys(p, q):
n = p*q
phi_n = (p-1)*(q-1)
e = 65537 # 公钥选择一个常用的质数作为指数
d = modinv(e, phi_n) # 私钥计算模n下e的逆元
public_key = (e, n)
private_key = (d, n)
return public_key, private_key
# 加密函数
def encrypt(message, public_key):
message_num = int.from_bytes(message.encode(), 'big')
ciphertext = pow(message_num, public_key[0], public_key[1])
return ciphertext.to_bytes(math.ceil(ciphertext.bit_length() / 8), byteorder='big')
# 解密函数
def decrypt(ciphertext, private_key):
ciphertext_num = int.from_bytes(ciphertext, 'big')
plaintext_num = pow(ciphertext_num, private_key[0], private_key[1])
return plaintext_num.to_bytes(math.ceil(plaintext_num.bit_length() / 8), byteorder='big').decode()
# 示例使用
if __name__ == "__main__":
p = 61
q = 53
public_key, private_key = generate_keys(p, q)
message = "Hello, RSA!"
encrypted = encrypt(message.encode(), public_key)
decrypted = decrypt(encrypted, private_key)
print(f"原始消息: {message}")
print(f"加密后: {decrypted} (应与原始消息一致)")
```
请注意,这个示例使用了第三方库`Crypto.Util.number`,你需要先安装它才能运行代码。此外,实际应用中需要考虑安全性和性能优化,例如使用大素数和高效的大整数运算。
阅读全文
相关推荐
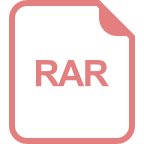
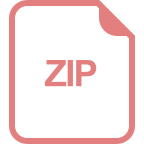
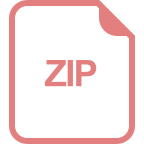

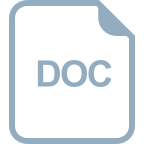



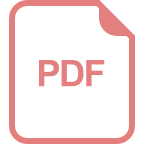
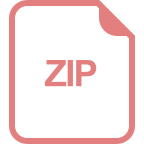
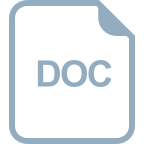
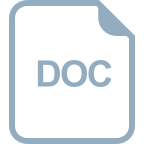






