贪心算法活动选择问题C语言或者Python代码
时间: 2024-12-23 07:27:26 浏览: 24
贪心算法是一种解决问题的策略,它在每一步都采取当前状态下最好、最直接的选择,希望这样的局部最优解能够累积成全局最优解。对于活动选择问题,也称为任务调度或课程表问题,通常涉及在一个有限的时间窗口内选择一系列互斥的任务。
以下是用Python和C语言简单实现的活动选择问题(即0-1背包问题简化版)的贪心策略:
**Python示例:**
```python
def activity_selection(events):
events.sort(key=lambda x: x[1]) # 按结束时间升序排列
selected = [events[0]]
for i in range(1, len(events)):
if events[i][0] > selected[-1][1]:
selected.append(events[i])
return selected
# 示例事件列表,每个元素是一个元组 (开始时间, 结束时间)
events = [(1, 3), (2, 4), (3, 5), (6, 7)]
print(activity_selection(events))
```
**C语言示例:**
```c
#include <stdio.h>
#include <stdlib.h>
// 比较结构体按结束时间排序
typedef struct {
int start;
int end;
} Event;
int compare(const void *a, const void *b) {
Event *ea = (Event *)a, *eb = (Event *)b;
return ea->end - eb->end;
}
void activity_selection(Event *events, int n) {
qsort(events, n, sizeof(Event), compare);
Event *selected[] = {events};
for (int i = 1; i < n; ++i) {
if (events[i].start > selected[0]->end) {
selected[0] = &events[i];
}
}
printf("Selected activities:\n");
for (int i = 0; i <= n; ++i) {
printf("(%d,%d)\n", selected[i]->start, selected[i]->end);
}
}
int main() {
Event events[] = {{1, 3}, {2, 4}, {3, 5}, {6, 7}};
activity_selection(events, sizeof(events) / sizeof(events[0]));
return 0;
}
```
这两个示例都是基于假设所有活动在同一时间段内是独立的,并且只考虑了时间线上的顺序。实际应用中可能需要更复杂的策略。
阅读全文
相关推荐
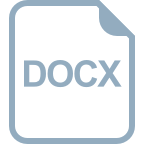
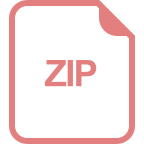
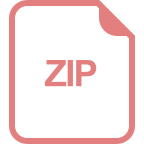
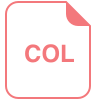
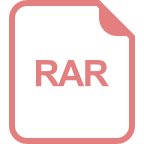
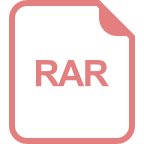
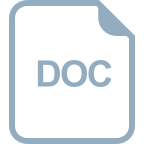
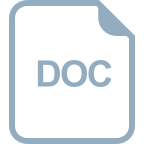
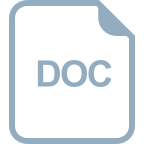
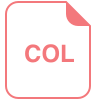
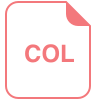
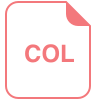

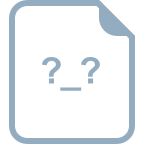
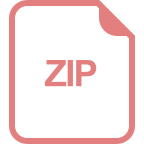
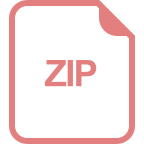
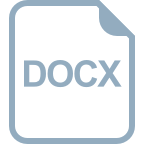