pyqt5mysql登录验证_pyqt5 设计简单登陆界面,含记住密码功能
时间: 2023-06-19 16:05:00 浏览: 77
以下是一个简单的 PyQT5 登录界面,包括记住密码的功能和基于 MySQL 的验证:
```python
import sys
from PyQt5.QtWidgets import QApplication, QWidget, QLabel, QLineEdit, QPushButton, QHBoxLayout, QVBoxLayout, QMessageBox
import mysql.connector
class Login(QWidget):
def __init__(self):
super().__init__()
# 初始化数据库连接
self.cnx = mysql.connector.connect(user='root', password='password', host='localhost', database='testdb')
self.cursor = self.cnx.cursor()
# 初始化 UI
self.initUI()
def initUI(self):
# 创建组件
self.label_username = QLabel('Username')
self.label_password = QLabel('Password')
self.edit_username = QLineEdit()
self.edit_password = QLineEdit()
self.edit_password.setEchoMode(QLineEdit.Password)
self.btn_login = QPushButton('Login')
self.btn_login.clicked.connect(self.login)
self.btn_cancel = QPushButton('Cancel')
self.btn_cancel.clicked.connect(self.close)
self.check_remember = QCheckBox('Remember me')
self.check_remember.setChecked(True) # 默认选中
# 创建布局
hbox_username = QHBoxLayout()
hbox_username.addWidget(self.label_username)
hbox_username.addWidget(self.edit_username)
hbox_password = QHBoxLayout()
hbox_password.addWidget(self.label_password)
hbox_password.addWidget(self.edit_password)
hbox_buttons = QHBoxLayout()
hbox_buttons.addWidget(self.btn_login)
hbox_buttons.addWidget(self.btn_cancel)
vbox = QVBoxLayout()
vbox.addLayout(hbox_username)
vbox.addLayout(hbox_password)
vbox.addWidget(self.check_remember)
vbox.addLayout(hbox_buttons)
# 设置窗口属性
self.setLayout(vbox)
self.setGeometry(300, 300, 300, 150)
self.setWindowTitle('Login')
# 读取记住密码的数据
settings = QSettings('myapp.ini', QSettings.IniFormat)
self.edit_username.setText(settings.value('username', ''))
self.edit_password.setText(settings.value('password', ''))
def closeEvent(self, event):
# 保存记住密码的数据
settings = QSettings('myapp.ini', QSettings.IniFormat)
if self.check_remember.isChecked():
settings.setValue('username', self.edit_username.text())
settings.setValue('password', self.edit_password.text())
else:
settings.remove('username')
settings.remove('password')
def login(self):
# 从数据库中验证用户名和密码
username = self.edit_username.text()
password = self.edit_password.text()
query = "SELECT * FROM users WHERE username=%s AND password=%s"
self.cursor.execute(query, (username, password))
result = self.cursor.fetchone()
if result:
QMessageBox.information(self, 'Success', 'Login successful')
else:
QMessageBox.warning(self, 'Error', 'Invalid username or password')
if __name__ == '__main__':
app = QApplication(sys.argv)
login = Login()
login.show()
sys.exit(app.exec_())
```
在这个例子中,我们使用了 MySQL 数据库进行验证,用户名和密码存储在一个名为 `users` 的表中。你需要将 `user`、`password`、`host` 和 `database` 更改为你的 MySQL 连接信息,并且需要确保在 MySQL 数据库中有一个名为 `users` 的表。
相关推荐
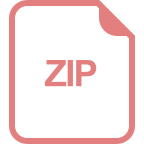
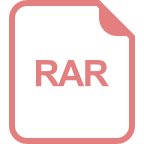
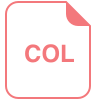
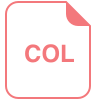
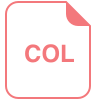
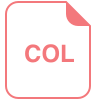
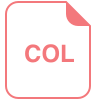









