用python写一个股票程序
时间: 2023-04-07 21:02:59 浏览: 77
好的,我可以回答这个问题。使用Python编写股票程序可以使用一些第三方库,例如pandas、numpy和matplotlib等。可以使用pandas获取股票数据,使用numpy进行计算和分析,使用matplotlib进行可视化。同时,还可以使用一些机器学习算法来预测股票价格。需要注意的是,股票市场是非常复杂和不稳定的,程序的准确性和可靠性需要经过充分的测试和验证。
相关问题
用python写一个股票买卖程序
由于股票买卖程序涉及到实时数据和交易,需要使用专业的API和接口,这里提供一个基础的股票买卖程序框架,仅供参考。
```
# 导入必要的库
import time
import random
# 定义股票类
class Stock:
def __init__(self, name, price):
self.name = name
self.price = price
# 获取股票价格
def get_price(self):
# 这里使用随机数生成价格,实际情况需要使用API获取实时数据
self.price = round(random.uniform(10, 100), 2)
return self.price
# 定义账户类
class Account:
def __init__(self, username, balance):
self.username = username
self.balance = balance
# 显示账户余额
def show_balance(self):
print("账户余额为:%.2f" % self.balance)
# 买入股票
def buy_stock(self, stock, amount):
price = stock.get_price()
total_price = price * amount
if self.balance >= total_price:
self.balance -= total_price
print("买入股票%s,数量为%d,花费%.2f元" % (stock.name, amount, total_price))
else:
print("账户余额不足,无法购买股票")
# 卖出股票
def sell_stock(self, stock, amount):
price = stock.get_price()
total_price = price * amount
self.balance += total_price
print("卖出股票%s,数量为%d,获得%.2f元" % (stock.name, amount, total_price))
# 测试程序
if __name__ == '__main__':
# 创建账户和股票
account = Account("Alice", 1000)
stock = Stock("AAPL", 0)
# 购买股票
account.show_balance()
account.buy_stock(stock, 10)
account.show_balance()
# 卖出股票
account.sell_stock(stock, 5)
account.show_balance()
# 循环更新股票价格
while True:
stock.get_price()
time.sleep(1)
```
这个程序使用了一个简单的股票类和账户类,通过调用股票类的get_price()方法获取股票价格,通过账户类的buy_stock()和sell_stock()方法进行买卖操作。程序还包括一个循环更新股票价格的代码,可以实时反映股票价格的变化。
需要注意的是,这个程序仅用于演示,实际的股票买卖程序需要使用专业的API和接口,且需要考虑到实时性、稳定性和安全性等问题。
用Python写一个股票预测
股票预测是一项非常复杂的任务,需要深入的数据分析和机器学习技术。这里提供一个基础的股票预测的 Python 代码,介绍基本的数据处理和模型构建流程。
首先,我们需要导入需要的库。
```python
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
from sklearn.preprocessing import MinMaxScaler
from keras.models import Sequential
from keras.layers import Dense, LSTM, Dropout
```
接下来,我们需要获取历史股票数据。这里以雅虎财经为例,使用 pandas 库获取股票历史数据并进行可视化。
```python
df = pd.read_csv('AAPL.csv')
df.head()
plt.figure(figsize=(16,8))
plt.title('Close Price History')
plt.plot(df['Close'])
plt.xlabel('Date', fontsize=18)
plt.ylabel('Close Price USD ($)', fontsize=18)
plt.show()
```
接下来,我们需要对数据进行处理,包括归一化和分割训练集和测试集。这里采用 MinMaxScaler 进行数据归一化和 80/20 的比例分割训练集和测试集。
```python
# Create a new dataframe with only the 'Close' column
data = df.filter(['Close'])
# Convert the dataframe to a numpy array
dataset = data.values
# Get the number of rows to train the model on
training_data_len = int(np.ceil( len(dataset) * 0.8 ))
# Scale the data
scaler = MinMaxScaler(feature_range=(0, 1))
scaled_data = scaler.fit_transform(dataset)
# Create the training data set
train_data = scaled_data[0:training_data_len, :]
# Split the data into x_train and y_train data sets
x_train = []
y_train = []
for i in range(60, len(train_data)):
x_train.append(train_data[i-60:i, 0])
y_train.append(train_data[i, 0])
# Convert the x_train and y_train to numpy arrays
x_train, y_train = np.array(x_train), np.array(y_train)
# Reshape the data
x_train = np.reshape(x_train, (x_train.shape[0], x_train.shape[1], 1))
```
接下来,我们可以开始构建 LSTM 模型,并使用训练集进行训练。这里使用了 2 层 LSTM 和 2 层 Dropout,最后添加一个全连接层作为输出层。模型的优化器采用 Adam。
```python
# Build the LSTM model
model = Sequential()
model.add(LSTM(units=50, return_sequences=True, input_shape=(x_train.shape[1], 1)))
model.add(Dropout(0.2))
model.add(LSTM(units=50, return_sequences=True))
model.add(Dropout(0.2))
model.add(LSTM(units=50))
model.add(Dropout(0.2))
model.add(Dense(units=1))
# Compile the model
model.compile(optimizer='adam', loss='mean_squared_error')
# Train the model
model.fit(x_train, y_train, epochs=50, batch_size=32)
```
最后,我们使用测试集进行预测,并可视化预测结果。
```python
# Create the testing data set
test_data = scaled_data[training_data_len - 60: , :]
# Create the x_test and y_test data sets
x_test = []
y_test = dataset[training_data_len:, :]
for i in range(60, len(test_data)):
x_test.append(test_data[i-60:i, 0])
# Convert the data to a numpy array
x_test = np.array(x_test)
# Reshape the data
x_test = np.reshape(x_test, (x_test.shape[0], x_test.shape[1], 1))
# Get the models predicted price values
predictions = model.predict(x_test)
predictions = scaler.inverse_transform(predictions)
# Plot the data
train = data[:training_data_len]
valid = data[training_data_len:]
valid['Predictions'] = predictions
plt.figure(figsize=(16,8))
plt.title('Model')
plt.xlabel('Date', fontsize=18)
plt.ylabel('Close Price USD ($)', fontsize=18)
plt.plot(train['Close'])
plt.plot(valid[['Close', 'Predictions']])
plt.legend(['Train', 'Val', 'Predictions'], loc='lower right')
plt.show()
```
这就是一个基础的股票预测的 Python 代码。需要注意的是,股票预测是一项复杂的任务,以上代码只是基础的流程,具体的预测效果需要根据具体情况进行优化和调整。
相关推荐
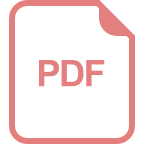
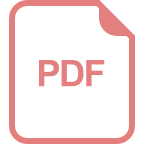












