写一个简单的3D烟花代码
时间: 2023-12-01 21:05:00 浏览: 69
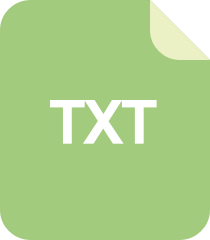
烟花源代码

以下是一个简单的基于Three.js的3D烟花代码:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>3D Fireworks</title>
<style>
body {
margin: 0;
overflow: hidden;
}
canvas {
width: 100%;
height: 100%;
}
</style>
</head>
<body>
<script src="https://cdnjs.cloudflare.com/ajax/libs/three.js/r128/three.min.js"></script>
<script>
// 初始化场景、相机、渲染器
const scene = new THREE.Scene();
const camera = new THREE.PerspectiveCamera(75, window.innerWidth / window.innerHeight, 0.1, 1000);
const renderer = new THREE.WebGLRenderer({ antialias: true });
renderer.setSize(window.innerWidth, window.innerHeight);
document.body.appendChild(renderer.domElement);
// 创建烟花
function createFirework() {
const firework = new THREE.Group();
const colors = [0xff0000, 0x00ff00, 0x0000ff, 0xffff00, 0xff00ff, 0x00ffff];
const color = colors[Math.floor(Math.random() * colors.length)];
const radius = Math.random() * 1 + 0.5;
// 创建中心球体
const centerGeometry = new THREE.SphereGeometry(radius, 10, 10);
const centerMaterial = new THREE.MeshBasicMaterial({ color: color });
const centerSphere = new THREE.Mesh(centerGeometry, centerMaterial);
firework.add(centerSphere);
// 创建爆炸效果
for (let i = 0; i < 50; i++) {
const particleGeometry = new THREE.SphereGeometry(0.1, 10, 10);
const particleMaterial = new THREE.MeshBasicMaterial({ color: color });
const particle = new THREE.Mesh(particleGeometry, particleMaterial);
particle.position.set(
centerSphere.position.x + (Math.random() - 0.5) * 5,
centerSphere.position.y + (Math.random() - 0.5) * 5,
centerSphere.position.z + (Math.random() - 0.5) * 5
);
firework.add(particle);
// 添加动画效果
const targetPosition = new THREE.Vector3(
(Math.random() - 0.5) * 30,
(Math.random() - 0.5) * 30,
(Math.random() - 0.5) * 30
);
const tween = new TWEEN.Tween(particle.position)
.to(targetPosition, 1000)
.easing(TWEEN.Easing.Quadratic.Out)
.start();
}
// 添加动画效果
const targetScale = new THREE.Vector3(0, 0, 0);
const tween = new TWEEN.Tween(centerSphere.scale)
.to(targetScale, 1000)
.easing(TWEEN.Easing.Quadratic.Out)
.onComplete(() => {
scene.remove(firework);
})
.start();
scene.add(firework);
}
// 渲染场景
function render() {
requestAnimationFrame(render);
TWEEN.update();
renderer.render(scene, camera);
}
// 初始化烟花
setInterval(createFirework, 1000);
// 调整窗口大小
window.addEventListener('resize', () => {
renderer.setSize(window.innerWidth, window.innerHeight);
camera.aspect = window.innerWidth / window.innerHeight;
camera.updateProjectionMatrix();
});
// 设置相机位置
camera.position.z = 50;
// 开始渲染场景
render();
</script>
</body>
</html>
```
该代码会在场景中随机创建不同颜色和大小的烟花,并添加动画效果。你可以在浏览器中打开该文件,看到烟花的效果。注意,该代码需要在服务器环境下才能正常运行。
阅读全文
相关推荐
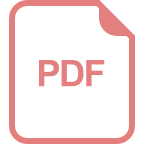
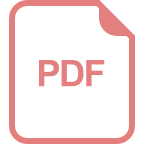
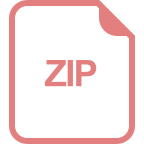
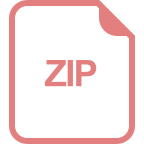
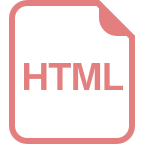
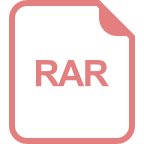
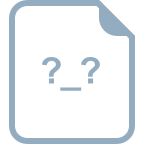
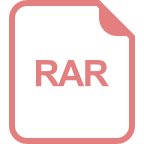
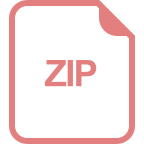
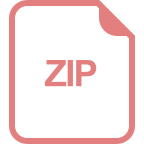
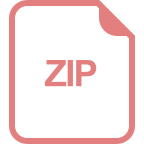



