一个具有三个成员的的结构体数组,给我一个根据第三个成员的大小来给数组排序的算法,c++
时间: 2024-12-18 11:31:25 浏览: 5
在C++中,你可以使用STL库中的`std::sort`函数结合自定义比较函数来对结构体数组按照第三个成员进行排序。假设我们有一个名为`MyStruct`的结构体,它有三个成员:`name`, `age`, 和 `score`。下面是一个简单的示例:
```cpp
#include <iostream>
#include <algorithm> // 包含sort函数
using namespace std;
// 假设 MyStruct 结构如下
struct MyStruct {
string name;
int age;
float score;
};
// 定义一个比较函数用于结构体数组排序,这里按照score降序
bool compare(const MyStruct& a, const MyStruct& b) {
return a.score > b.score; // 如果需要升序,则返回a.score < b.score;
}
void sortArrayByScore(MyStruct arr[], int size) {
if (size > 0) {
sort(arr, arr + size, compare); // 使用自定义比较函数对数组进行排序
}
}
int main() {
MyStruct students[3] = {{"Tom", 20, 85.5}, {"Jerry", 22, 90.0}, {"Spike", 18, 78.0}};
int n = sizeof(students) / sizeof(students[0]);
cout << "Before sorting:\n";
for (int i = 0; i < n; ++i) {
cout << students[i].name << ", " << students[i].age << ", " << students[i].score << endl;
}
sortArrayByScore(students, n);
cout << "\nAfter sorting by score in descending order:\n";
for (int i = 0; i < n; ++i) {
cout << students[i].name << ", " << students[i].age << ", " << students[i].score << endl;
}
return 0;
}
```
在这个例子中,`compare`函数负责比较两个结构体的score字段。运行这个程序,你会看到数组按score值降序排列。
阅读全文
相关推荐
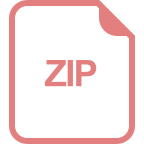
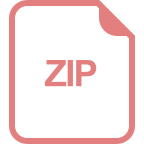
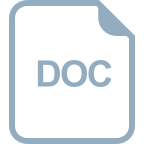
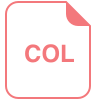

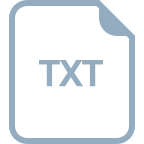
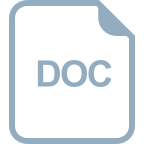
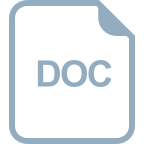
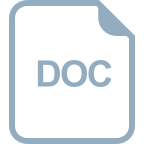
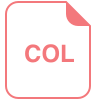
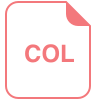
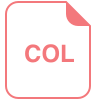
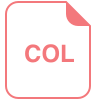
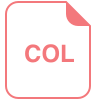
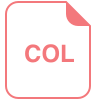
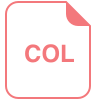
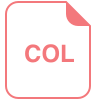

