vector容器结构体排序
时间: 2024-12-26 19:11:09 浏览: 4
Vector是C++标准库中的动态数组,它提供了一种线性的内存布局,可以动态调整大小。当涉及到排序vector容器中的元素时,有两种常用的方式:
1. **内置排序功能**:`std::sort()`函数可以用于对vector内的元素进行排序。你可以直接传递vector的起始迭代器和结束迭代器给这个函数,例如:
```cpp
std::sort(your_vector.begin(), your_vector.end());
```
如果需要自定义比较规则,可以提供一个比较函数作为第三个参数。
2. **使用STL算法**:如`std::stable_sort()`或`std::partial_sort()`等,它们提供了更细粒度的控制。
如果你想在vector内部按照某个成员变量进行排序,可以用lambda表达式或函数指针来指定排序依据:
```cpp
std::sort(your_vector.begin(), your_vector.end(), [](const YourClass& a, const YourClass& b) {
return a.your_member < b.your_member;
});
```
这里`your_member`是你想用来排序的类的成员变量。
相关问题
vector结构体排序
以下是使用lambda表达式对vector结构体进行排序的示例代码:
```cpp
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
struct Student {
string name;
int score;
};
int main() {
vector<Student> students = {{"Tom", 80}, {"Jerry", 90}, {"Alice", 70}};
// 按照分数降序排序
sort(students.begin(), students.end(), [](const Student& a, const Student& b) {
return a.score > b.score;
});
// 输出排序结果
for (const auto& student : students) {
cout << student.name << " " << student.score << endl;
}
return 0;
}
```
上述代码中,我们定义了一个结构体`Student`,包含学生姓名和分数两个成员变量。然后我们定义了一个`vector`类型的容器`students`,其中存储了三个学生的信息。接着,我们使用`sort`函数对`students`进行排序,排序的依据是学生的分数,按照降序排列。最后,我们遍历排序后的`students`容器,输出每个学生的姓名和分数。
c++结构体排序练习题
在C++中,结构体排序练习通常涉及到如何使用标准库中的算法对包含自定义数据类型的结构体数组或容器进行排序。这里我们可以举一个简单的例子,假设有一个名为`Student`的结构体,包含`name`和`age`两个成员:
```cpp
struct Student {
std::string name;
int age;
};
```
你可以用以下几种方法对`Student`结构体数组进行排序:
1. **直接排序:**如果年龄是排序的主要依据,你可以定义一个比较函数(`compare`),然后使用`std::sort`函数:
```cpp
bool compareStudents(const Student& s1, const Student& s2) {
return s1.age < s2.age;
}
int main() {
Student students[] = {{"Alice", 20}, {"Bob", 18}, {"Charlie", 22}};
std::sort(students, students + sizeof(students) / sizeof(students), compareStudents);
// 现在students数组按年龄升序排列
}
```
2. **使用STL算法:**如果你的结构体已经实现了`<`运算符,那么可以直接使用`std::stable_sort`:
```cpp
bool studentLess(const Student& s1, const Student& s2) {
return s1.age < s2.age;
}
int main() {
std::vector<Student> students = {{"Alice", 20}, {"Bob", 18}, {"Charlie", 22}};
std::stable_sort(students.begin(), students.end(), studentLess);
}
```
3. **自定义比较器(C++11及以上):**也可以使用lambda表达式来创建一个可传递的比较器:
```cpp
int main() {
std::vector<Student> students = {{"Alice", 20}, {"Bob", 18}, {"Charlie", 22}};
std::sort(students.begin(), students.end(), [](const Student& s1, const Student& s2) {
return s1.age < s2.age;
});
}
```
阅读全文
相关推荐
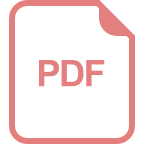
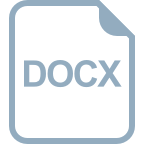
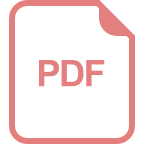













