<template> <div> <div v-for="(item, index) in items" :key="index" class="row"> <button :disabled="!start || !item.checked" @click="clickButton(index, item.delay)" :class="{ active: item.active }" > Button {{ index + 1 }} <span v-if="item.active" class="click-animation"></span> </button> <input type="checkbox" v-model="item.checked" :disabled="!start"/> </div> <button :class="{ start: start, stop: !start }" @click="startStop">{{ start ? 'Stop' : 'Start' }}</button> </div> </template> <script> export default { data () { return { items: [ {checked: false, delay: 100, active: false}, {checked: false, delay: 200, active: false}, {checked: false, delay: 300, active: false}, {checked: false, delay: 400, active: false} ], start: true, intervalIds: [] } }, methods: { clickButton (index, delay) { this.items[index].active = true setTimeout(() => { console.log(`Clicked button ${index + 1}`) this.items[index].active = false }, 100) if (this.items[2].checked) { setTimeout(() => { console.log('Clicked button 1 of the third row') }, delay) setTimeout(() => { console.log('Clicked button 3 of the third row') }, delay + 300) } }, startStop () { this.start = !this.start if (this.start) { this.intervalIds.forEach((id) => clearInterval(id)) } else { this.intervalIds = this.items.map((item, index) => { if (item.checked) { return setInterval(() => { this.clickButton(index, item.delay) }, item.delay) } else { return null } }) } } } } </script>如何将第一行按钮的挨骂也改成点击开始和每0.1秒执行一下
时间: 2024-01-15 15:02:48 浏览: 30
您可以在模板中将第一行按钮的文本从 "Button 1" 更改为 "开始",然后将其点击事件绑定到一个新的方法 `startClick` 上。在 `startClick` 方法中,您可以使用 `setInterval` 方法来执行 `clickButton` 方法,以便每隔 0.1 秒点击一下按钮。以下是修改后的代码示例:
```
<template>
<div>
<div v-for="(item, index) in items" :key="index" class="row">
<button
v-if="index === 0"
:disabled="!start || !item.checked"
@click="startClick"
:class="{ active: item.active }"
>
{{ start ? '开始' : '停止' }}
<span v-if="item.active" class="click-animation"></span>
</button>
<button
v-else
:disabled="!start || !item.checked"
@click="clickButton(index, item.delay)"
:class="{ active: item.active }"
>
Button {{ index }}
<span v-if="item.active" class="click-animation"></span>
</button>
<input type="checkbox" v-model="item.checked" :disabled="!start"/>
</div>
<button :class="{ start: start, stop: !start }" @click="startStop">{{ start ? 'Stop' : 'Start' }}</button>
</div>
</template>
<script>
export default {
data () {
return {
items: [
{checked: false, delay: 100, active: false},
{checked: false, delay: 200, active: false},
{checked: false, delay: 300, active: false},
{checked: false, delay: 400, active: false}
],
start: true,
intervalIds: []
}
},
methods: {
clickButton (index, delay) {
this.items[index].active = true
setTimeout(() => {
console.log(`Clicked button ${index}`)
this.items[index].active = false
}, 100)
if (this.items[2].checked) {
setTimeout(() => {
console.log('Clicked button 1 of the third row')
}, delay)
setTimeout(() => {
console.log('Clicked button 3 of the third row')
}, delay + 300)
}
},
startClick () {
if (this.start) {
this.start = false
this.intervalIds.forEach((id) => clearInterval(id))
} else {
this.start = true
this.intervalIds = this.items.map((item, index) => {
if (item.checked) {
return setInterval(() => {
this.clickButton(index, item.delay)
}, 100)
} else {
return null
}
})
}
},
startStop () {
this.start = !this.start
if (this.start) {
this.intervalIds.forEach((id) => clearInterval(id))
} else {
this.intervalIds = this.items.map((item, index) => {
if (item.checked) {
return setInterval(() => {
this.clickButton(index, item.delay)
}, item.delay)
} else {
return null
}
})
}
}
}
}
</script>
```
相关推荐
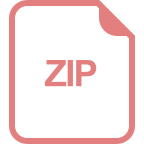
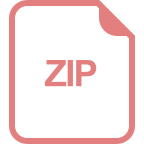














