nlp=StanfordCoreNLP()这句代码是用来干什么的
时间: 2024-02-28 20:55:53 浏览: 92
这句代码是用来创建一个StanfordCoreNLP对象的实例,用于执行自然语言处理(Natural Language Processing,NLP)任务。StanfordCoreNLP是由斯坦福大学自然语言处理组开发的一款NLP工具包,该工具包提供了一系列的NLP功能,例如分词、词性标注、命名实体识别、句法分析、情感分析等。
在创建StanfordCoreNLP对象实例时,可以指定一些配置参数,例如要使用的分词器、词性标注器、命名实体识别器、句法分析器等。具体的配置参数可以在创建对象实例时通过参数进行设置,例如:
```
nlp = StanfordCoreNLP('http://localhost:9000', language='en', timeout=30000)
```
上述代码创建了一个StanfordCoreNLP对象实例,指定了使用英文分词器和词性标注器,超时时间为30秒。创建对象实例后,可以调用其提供的各种方法来执行不同的NLP任务,例如:
```
text = 'John likes to play soccer.'
tokens = nlp.word_tokenize(text)
print(tokens)
```
上述代码使用StanfordCoreNLP对象实例的word_tokenize方法对输入文本进行分词,返回分词结果。
相关问题
nlp = StanfordCoreNLP(r'D:\anaconda\envs\pytorch\Lib\site-packages\stanford-corenlp-full-2018-02-27', lang='zh')
这是一行Python代码,使用StanfordCoreNLP库创建了一个名为nlp的对象,指定了Stanford CoreNLP工具的安装路径,并设置了语言为中文。可以使用该对象对中文文本进行自然语言处理(NLP)操作,例如分词、词性标注、命名实体识别、依存句法分析等。需要注意的是,StanfordCoreNLP库需要依赖Java环境,在使用前需要确保已经正确安装了Java并配置了环境变量。
import nltk.corpus import pandas as pd import re import matplotlib.pyplot as plt import seaborn as sns from stanfordcorenlp import StanfordCoreNLP # 导入数据 df = pd.read_csv('D:/file document/desktop/语料库大作业/Tweets.csv', usecols=['airline_sentiment', 'text']) def sentiment(x): if x == 'positive': return 1 elif x == 'negative': return -1 else: return 0 from nltk.corpus import stopwords from nltk.stem import SnowballStemmer from nltk.tokenize import RegexpTokenizer # 去除停用词 stopwords = nltk.corpus.stopwords.words('english') # 词还原 stemmer = SnowballStemmer('english') # 分词 tokenizer = RegexpTokenizer(r'\w+') # As this dataset is fetched from twitter so it has lots of people tag in tweets # we will remove them tags = r"@\w*" def preprocess_text(sentence, stem=False): # 去除text中一些影响文本分析的标签 sentence = [re.sub(tags, "", sentence)] text = [] for word in sentence: if word not in stopwords: if stem: text.append(stemmer.stem(word).lower()) else: text.append(word.lower()) return tokenizer.tokenize(" ".join(text)) # 将用preprocess_text() 函数处理后的text列保存回原始 DataFrame 的 text 列中 df['text'] = df['text'].map(preprocess_text) output_file = 'D:/file document/desktop/语料库大作业/output2.csv' # 输出文件路径 nlp = StanfordCoreNLP(r"D:/AppData/stanfordnlp", lang="en") # 定义函数,用于对指定文本进行依存句法分析 def dependency_parse(sentence): result = nlp.dependency_parse(sentence) return result # 对某一列进行依存句法分析,并将结果保存到新的一列中 df['dependency_parse'] = df['text'].apply(lambda x: dependency_parse(" ".join(x))) # 将结果保存到输出文件中 df.to_csv(output_file, index=False) nlp.close()优化这段代码
这段代码的主要任务是对一份推特数据集进行预处理,并对推特文本进行依存句法分析。以下是对该代码进行优化的一些建议:
1. 对导入的模块进行适当的组织和注释,以增加代码的可读性。
2. 将数据集的路径和输出文件的路径定义为变量,以方便修改。
3. 在进行依存句法分析之前,应该先将文本数据转换为字符串类型,以免引起类型错误。
4. 将StanfordCoreNLP的连接和关闭放在 with 语句块中,以确保它在使用后自动关闭连接。
5. 改进 preprocess_text() 函数,使其更加健壮和可读。
6. 使用 pandas 的 apply() 函数来遍历数据集,而不是使用 for 循环。
7. 将依存句法分析的结果转换为字符串类型,以便于保存到输出文件中。
下面是针对上述优化建议修改后的代码:
```
import re
import nltk
import pandas as pd
from nltk.corpus import stopwords
from nltk.stem import SnowballStemmer
from nltk.tokenize import RegexpTokenizer
from stanfordcorenlp import StanfordCoreNLP
# Define file paths
input_file = 'D:/file document/desktop/语料库大作业/Tweets.csv'
output_file = 'D:/file document/desktop/语料库大作业/output2.csv'
# Define sentiment function
def sentiment(x):
if x == 'positive':
return 1
elif x == 'negative':
return -1
else:
return 0
# Define preprocessing functions
stopwords = set(stopwords.words('english'))
stemmer = SnowballStemmer('english')
tokenizer = RegexpTokenizer(r'\w+')
tags = r"@\w*"
def preprocess_text(sentence, stem=False):
sentence = re.sub(tags, "", sentence)
words = tokenizer.tokenize(sentence)
words = [word.lower() for word in words if word.lower() not in stopwords]
if stem:
words = [stemmer.stem(word) for word in words]
return words
# Load data
df = pd.read_csv(input_file, usecols=['airline_sentiment', 'text'])
# Preprocess text
df['text'] = df['text'].apply(lambda x: preprocess_text(x))
# Connect to StanfordCoreNLP
with StanfordCoreNLP(r"D:/AppData/stanfordnlp", lang="en") as nlp:
# Define function for dependency parsing
def dependency_parse(sentence):
result = nlp.dependency_parse(str(sentence))
return str(result)
# Apply dependency parsing to text column and save results to new column
df['dependency_parse'] = df['text'].apply(lambda x: dependency_parse(x))
# Save preprocessed data to output file
df.to_csv(output_file, index=False)
```
在优化后的代码中,我们将数据集的路径和输出文件的路径定义为变量,以方便修改和维护。同时,我们对代码进行了适当的注释和组织,以增加代码的可读性。我们也改进了 preprocess_text() 函数,使其更加健壮和可读。最后,我们还使用了 with 语句块来管理 StanfordCoreNLP 的连接和关闭,以确保它在使用后自动关闭连接。
阅读全文
相关推荐
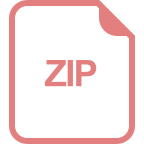
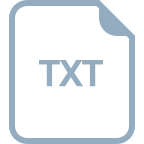
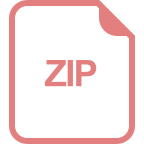
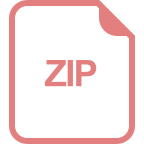
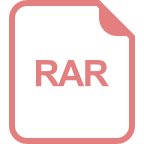
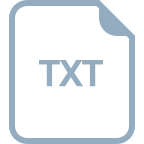
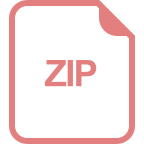
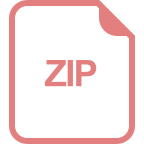
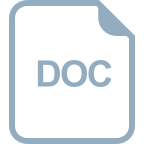
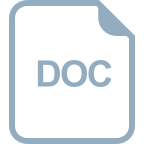


