QTimer::singleShot示例
时间: 2024-03-30 20:30:09 浏览: 52
以下是一个使用QTimer::singleShot函数的示例:
```cpp
#include <QApplication>
#include <QTimer>
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
// 创建一个定时器,延迟1秒后执行槽函数
QTimer::singleShot(1000, [](){
// 在这里编写你想要执行的代码
// 例如打印一条消息
qDebug() << "Hello, QTimer!";
});
return app.exec();
}
```
这个示例中,我们创建了一个定时器,使用QTimer::singleShot函数设置了一个1秒的延迟。在延迟结束后,定时器会触发槽函数,我们在槽函数中编写了打印一条消息的代码。当程序运行时,1秒后会在控制台输出"Hello, QTimer!"。
相关问题
QTimer::singleShot(
QTimer::singleShot是Qt框架中的一个静态函数,于在指定的时间间隔后执行一次特定的槽函数或者Lambda表达式。
该函数的原型如下:
```
void QTimer::singleShot(int msec, const QObject *receiver, const char *member)
```
其中,msec表示延迟的时间间隔(以毫秒为单位),receiver表示接收信号的对象,member表示要执行的槽函数的名称。
使用QTimer::singleShot可以实现一些延迟执行的功能,比如在一段时间后显示一个提示信息、延迟加载某个资源等。
示例代码:
```cpp
#include <QApplication>
#include <QTimer>
#include <QDebug>
class MyClass : public QObject
{
Q_OBJECT
public slots:
void mySlot()
{
qDebug() << "Delayed execution";
}
};
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
MyClass obj;
QTimer::singleShot(2000, &obj, SLOT(mySlot())); // 2秒后执行mySlot函数
return a.exec();
}
```
上述代码中,通过QTimer::singleShot函数实现了2秒后执行mySlot函数的功能。当时间到达时,会自动触发mySlot函数,并输出"Delayed execution"。
qtimer::singleshot如何关闭
要关闭 QTimer::singleShot,您可以使用其返回的 QTimer 对象的 stop() 方法。以下是一个示例:
```cpp
QTimer* timer = new QTimer(this);
connect(timer, &QTimer::timeout, [=]() {
// 定时器超时后执行的代码
});
timer->start(1000); // 启动定时器
// 在某个地方调用以下代码来关闭定时器
timer->stop();
delete timer;
```
在上面的示例中,我们首先创建了一个 QTimer 对象,并使用 connect() 函数连接了 timeout 信号与我们想要执行的槽函数。然后,我们通过调用 start() 方法启动定时器,设置了定时器的超时时间为 1000 毫秒。
要关闭定时器,我们调用 timer 的 stop() 方法来停止计时器,并使用 delete 关键字删除定时器对象。
请注意,这里使用的是 QTimer::singleShot 的变种,但关闭定时器的方法是相同的。
阅读全文
相关推荐
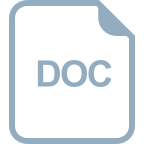
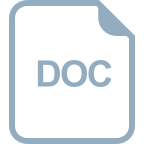
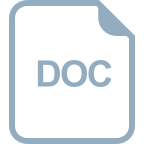













