请解决以上代码在Ubuntu运行中出现的以下问题:called object ‘pthread_create’ is not a function or function pointer pthread_create(&mole_threads[i], NULL, mole_thread, &i);
时间: 2023-08-18 15:08:12 浏览: 147
在Ubuntu运行中出现 "called object ‘pthread_create’ is not a function or function pointer" 的错误,通常是由于没有正确链接线程库引起的。为了解决这个问题,你可以在编译时添加 `-pthread` 选项来显式链接线程库。以下是修改后的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <pthread.h>
#include <stdbool.h>
#include <time.h>
#define SCREEN_WIDTH 40
#define SCREEN_HEIGHT 20
char screen[SCREEN_HEIGHT][SCREEN_WIDTH];
pthread_mutex_t screen_mutex = PTHREAD_MUTEX_INITIALIZER;
int score = 0;
typedef struct {
int x;
int y;
} Hole;
Hole holes[5];
void init_screen() {
for (int i = 0; i < SCREEN_HEIGHT; i++) {
for (int j = 0; j < SCREEN_WIDTH; j++) {
screen[i][j] = ' ';
}
}
}
void display_screen() {
system("clear");
for (int i = 0; i < SCREEN_HEIGHT; i++) {
for (int j = 0; j < SCREEN_WIDTH; j++) {
printf("%c", screen[i][j]);
}
printf("\n");
}
printf("Score: %d\n", score);
}
void update_screen(int x, int y, char character) {
pthread_mutex_lock(&screen_mutex);
screen[y][x] = character;
pthread_mutex_unlock(&screen_mutex);
}
void clear_screen(int x, int y) {
pthread_mutex_lock(&screen_mutex);
screen[y][x] = ' ';
pthread_mutex_unlock(&screen_mutex);
}
void* mole_thread(void* arg) {
int index = *(int*) arg;
srand(time(NULL) + index); // 根据线程索引设置随机种子
while (true) {
int x = rand() % SCREEN_WIDTH;
int y = rand() % SCREEN_HEIGHT;
update_screen(x, y, 'M');
usleep(500000); // 线程休眠0.5秒
clear_screen(x, y);
usleep(500000);
}
return NULL;
}
void* player_thread(void* arg) {
char input;
while (true) {
scanf(" %c", &input);
if (input == 'q') {
break;
}
if (input == 'h') {
pthread_mutex_lock(&screen_mutex);
for (int i = 0; i < 5; i++) {
if (screen[holes[i].y][holes[i].x] == 'M') {
score++;
clear_screen(holes[i].x, holes[i].y);
}
}
pthread_mutex_unlock(&screen_mutex);
}
}
return NULL;
}
int main() {
init_screen();
// 创建地鼠洞
srand(time(NULL));
for (int i = 0; i < 5; i++) {
holes[i].x = rand() % SCREEN_WIDTH;
holes[i].y = rand() % SCREEN_HEIGHT;
}
// 创建地鼠线程
pthread_t mole_threads[5];
for (int i = 0; i < 5; i++) {
pthread_create(&mole_threads[i], NULL, mole_thread, &i);
}
// 创建玩家线程
pthread_t player_thread_id;
pthread_create(&player_thread_id, NULL, player_thread, NULL);
// 游戏循环
while (true) {
display_screen();
usleep(100000); // 刷新屏幕间隔0.1秒
}
// 等待线程结束
for (int i = 0; i < 5; i++) {
pthread_join(mole_threads[i], NULL);
}
pthread_join(player_thread_id, NULL);
return 0;
}
```
在编译时使用以下命令来链接线程库:
```shell
gcc -o game game.c -pthread
```
这样应该就能解决 "called object ‘pthread_create’ is not a function or function pointer" 的问题了。
阅读全文
相关推荐
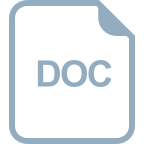
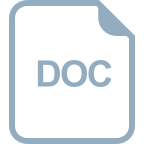
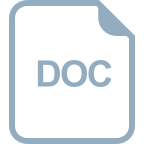















