写一个 C 语言的红黑树
时间: 2023-08-31 18:29:42 浏览: 54
### 回答1:
很高兴为你回答这个问题。C 语言的红黑树可以使用以下代码实现:#include <stdio.h>
#include <stdlib.h>struct node {
int data;
char color;
struct node *left, *right, *parent;
};struct node *newNode(int data)
{
struct node *n = (struct node*)malloc(sizeof(struct node));
n->data = data;
n->color = 'R';
n->left = NULL;
n->right = NULL;
n->parent = NULL; return n;
}void insert(struct node **root, int data)
{
struct node *n = newNode(data); if (*root == NULL)
{
*root = n;
}
else
{
struct node *temp = *root;
while (temp != NULL)
{
if (data > temp->data)
{
if (temp->right == NULL)
{
temp->right = n;
n->parent = temp;
break;
}
else
{
temp = temp->right;
}
}
else
{
if (temp->left == NULL)
{
temp->left = n;
n->parent = temp;
break;
}
else
{
temp = temp->left;
}
}
}
}
}void inorder(struct node *root)
{
if (root == NULL)
return; inorder(root->left);
printf("%d %c ", root->data, root->color);
inorder(root->right);
}int main()
{
struct node *root = NULL;
insert(&root, 10);
insert(&root, 5);
insert(&root, 15);
insert(&root, 3);
insert(&root, 8);
insert(&root, 20); inorder(root); return 0;
}
### 回答2:
红黑树是一种自平衡的二叉查找树,它通过在每个节点上增加一个额外的位来存储节点的颜色,使得树在插入、删除操作时始终保持平衡。
在C语言中实现红黑树主要包括以下几个步骤:
1. 定义节点结构体:
```c
typedef struct Node {
int data; // 存储节点数据
struct Node* parent; // 指向父节点
struct Node* left; // 指向左孩子节点
struct Node* right; // 指向右孩子节点
int isRed; // 是否为红节点,0表示黑色,1表示红色
} Node;
```
2. 定义红黑树结构体:
```c
typedef struct RedBlackTree {
Node* root; // 指向根节点
Node* nil; // 空节点的指针,表示叶子节点
} RedBlackTree;
```
3. 实现红黑树的插入操作:
```c
void insert(RedBlackTree* tree, int data) {
Node* newNode = createNode(data);
Node* x = tree->root;
Node* y = tree->nil;
while (x != tree->nil) {
y = x;
if (newNode->data < x->data) {
x = x->left;
}
else {
x = x->right;
}
}
newNode->parent = y;
if (y == tree->nil) {
tree->root = newNode;
}
else if (newNode->data < y->data) {
y->left = newNode;
}
else {
y->right = newNode;
}
newNode->left = tree->nil;
newNode->right = tree->nil;
newNode->isRed = 1;
insertFixup(tree, newNode);
}
void insertFixup(RedBlackTree* tree, Node* z) {
while (z->parent->isRed) {
if (z->parent == z->parent->parent->left) {
Node* y = z->parent->parent->right;
if (y->isRed) {
z->parent->isRed = 0;
y->isRed = 0;
z->parent->parent->isRed = 1;
z = z->parent->parent;
}
else {
if (z == z->parent->right) {
z = z->parent;
leftRotate(tree, z);
}
z->parent->isRed = 0;
z->parent->parent->isRed = 1;
rightRotate(tree, z->parent->parent);
}
}
else {
// 类似左右情况的对称处理
}
}
tree->root->isRed = 0;
}
```
4. 实现红黑树的旋转操作:
```c
void leftRotate(RedBlackTree* tree, Node* x) {
Node* y = x->right;
x->right = y->left;
if (y->left != tree->nil) {
y->left->parent = x;
}
y->parent = x->parent;
if (x->parent == tree->nil) {
tree->root = y;
}
else if (x == x->parent->left) {
x->parent->left = y;
}
else {
x->parent->right = y;
}
y->left = x;
x->parent = y;
}
void rightRotate(RedBlackTree* tree, Node* x) {
// 类似左旋的实现,进行对称处理
}
```
以上只是红黑树的基本实现,还可以继续完善其他操作,如删除、搜索等。在实际应用中,红黑树被广泛应用于数据结构和算法中,以提高效率和性能。
### 回答3:
红黑树(Red-Black Tree)是一种平衡二叉搜索树,它通过在每个节点上增加一个额外的存储位来表示节点的颜色,从而在维护了二叉搜索树特性的同时,也保持了树的平衡性。以下是用C语言实现红黑树的基本步骤:
1. 定义红黑树的结构体:
```
typedef struct Node {
int key;
int color;
struct Node *left, *right, *parent;
} Node;
typedef struct RedBlackTree {
Node *root;
Node *nil; // 表示树中的空节点
} RedBlackTree;
```
2. 实现红黑树的基本操作函数:
- 初始化函数:创建一个空的红黑树并初始化nil节点
```
RedBlackTree* createRedBlackTree() {
RedBlackTree *tree = (RedBlackTree*)malloc(sizeof(RedBlackTree));
tree->nil = (Node*)malloc(sizeof(Node));
tree->nil->color = 0; // 空节点颜色为黑色
tree->root = tree->nil; // 初始状态下根节点指向nil节点
return tree;
}
```
- 左旋操作:
```
void leftRotate(RedBlackTree *tree, Node *x) {
Node *y = x->right;
x->right = y->left;
if (y->left != tree->nil) {
y->left->parent = x;
}
y->parent = x->parent;
if (x->parent == tree->nil) {
tree->root = y;
} else if (x == x->parent->left) {
x->parent->left = y;
} else {
x->parent->right = y;
}
y->left = x;
x->parent = y;
}
```
- 右旋操作:
```
void rightRotate(RedBlackTree *tree, Node *y) {
Node *x = y->left;
y->left = x->right;
if (x->right != tree->nil) {
x->right->parent = y;
}
x->parent = y->parent;
if (y->parent == tree->nil) {
tree->root = x;
} else if (y == y->parent->left) {
y->parent->left = x;
} else {
y->parent->right = x;
}
x->right = y;
y->parent = x;
}
```
- 插入操作:
```
void insert(RedBlackTree *tree, int key) {
Node *z = (Node*)malloc(sizeof(Node));
z->key = key;
Node *y = tree->nil;
Node *x = tree->root;
while (x != tree->nil) {
y = x;
if (z->key < x->key) {
x = x->left;
} else {
x = x->right;
}
}
z->parent = y;
if (y == tree->nil) {
tree->root = z;
} else if (z->key < y->key) {
y->left = z;
} else {
y->right = z;
}
z->left = tree->nil;
z->right = tree->nil;
z->color = 1; // 插入节点为红色
insertFixup(tree, z);
}
```
- 插入操作修复函数:
```
void insertFixup(RedBlackTree *tree, Node *z) {
while (z->parent->color == 1) { // 当前节点的父节点是红色
if (z->parent == z->parent->parent->left) {
Node *y = z->parent->parent->right; // y是z的叔节点
if (y->color == 1) { // 叔节点是红色
z->parent->color = 0; // 将父节点颜色修改为黑色
y->color = 0; // 将叔节点颜色修改为黑色
z->parent->parent->color = 1; // 将祖父节点颜色修改为红色
z = z->parent->parent; // 已经保证了之后的黑高性质,从祖父节点继续修复
} else {
if (z == z->parent->right) {
z = z->parent;
leftRotate(tree, z);
}
z->parent->color = 0;
z->parent->parent->color = 1;
rightRotate(tree, z->parent->parent);
}
} else {
// 对称操作,左右调换即可
}
}
tree->root->color = 0; // 根节点为黑色
}
```
以上是红黑树的基本操作实现,包括了初始化函数、左旋和右旋操作、插入操作以及相应的修复函数。根据这些基本操作,可以实现其他常用的红黑树操作,如删除节点、搜索节点等。
相关推荐
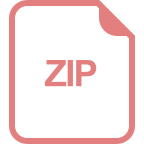
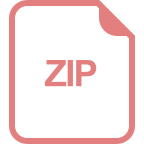






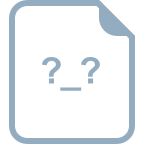
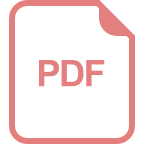
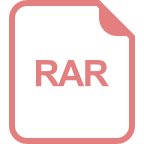
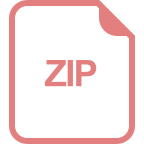
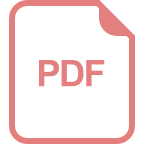
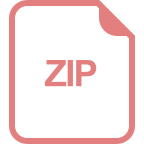
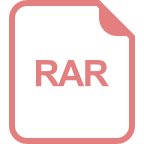
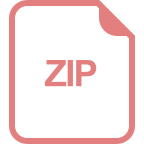