java链接MySQL数据库窗口输入用户密码
时间: 2023-10-08 22:03:51 浏览: 53
在Java中链接MySQL数据库时,可以通过使用JDBC API和MySQL Connector/J驱动程序来实现。如果需要在窗口输入用户密码,可以使用Java Swing中的JPasswordField组件。
以下是一个简单的示例代码,演示如何在窗口中输入MySQL数据库的用户名和密码:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
import java.sql.*;
public class MySQLLogin extends JFrame implements ActionListener {
JLabel userLabel = new JLabel("用户名:");
JTextField userTextField = new JTextField();
JLabel passwordLabel = new JLabel("密码:");
JPasswordField passwordField = new JPasswordField();
JButton loginButton = new JButton("登录");
public MySQLLogin() {
this.setTitle("MySQL登录");
this.setSize(300, 200);
this.setLayout(new GridLayout(3, 2));
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
this.add(userLabel);
this.add(userTextField);
this.add(passwordLabel);
this.add(passwordField);
this.add(loginButton);
loginButton.addActionListener(this);
this.setVisible(true);
}
public void actionPerformed(ActionEvent e) {
String user = userTextField.getText();
String password = new String(passwordField.getPassword());
try {
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", user, password);
System.out.println("连接成功");
conn.close();
} catch (SQLException ex) {
System.out.println("连接失败:" + ex.getMessage());
}
}
public static void main(String[] args) {
new MySQLLogin();
}
}
```
在上面的代码中,我们创建了一个MySQLLogin类,继承自JFrame,并实现了ActionListener接口。在构造函数中,我们创建了一个包含用户名、密码和登录按钮的窗口,并将登录按钮的ActionListener设置为this,以便在用户单击该按钮时调用actionPerformed方法。
在actionPerformed方法中,我们获取用户输入的用户名和密码,并使用DriverManager.getConnection方法链接到MySQL数据库。如果连接成功,我们将在控制台上输出“连接成功”,否则输出“连接失败”并显示异常信息。
请注意,在实际开发中,您应该将数据库连接信息存储在配置文件或环境变量中,以避免将敏感信息硬编码到代码中。
相关推荐
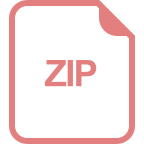
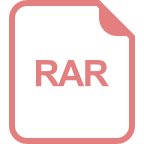
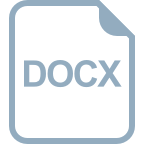
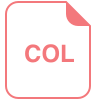
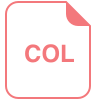
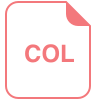
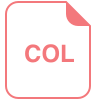
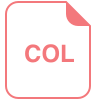









