@Service("AdminService") public class AdminServiceImpl implements AdminService { @Autowired private AdminDao adminDao; @Autowired private DocDao docDao; @Autowired private LogDao logDao; @Autowired private UserDao userDao; @Autowired private RoleDao roleDao; @Override public Admin login(String username, String password) { try { return adminDao.login(username, md5(password)); }catch (Exception e) { e.printStackTrace(); return null; } } @Override public Map<String, Integer> index() { Map<String,Integer> map = new HashMap<>(); Long templateNum = docDao.count(null); map.put("templateNum",templateNum.intValue()); List<Log> logs = logDao.selectAll(); int nowlogNum = 0; Date now = new Date(); for (Log log : logs) { Date date = log.getTime(); if(DateUtils.isSameDay(now, date)){ nowlogNum++; } } map.put("nowlogNum",nowlogNum); Long logNum= logDao.count(); map.put("logNum",logNum.intValue()); Long userNum = userDao.count(null); map.put("userNum",userNum.intValue()); Long roleNum = roleDao.count(); map.put("roleNum",roleNum.intValue()); return map; } public static String md5(String password) throws NoSuchAlgorithmException { MessageDigest md = MessageDigest.getInstance("MD5"); byte[] hash = md.digest(password.getBytes(StandardCharsets.UTF_8)); StringBuilder hexString = new StringBuilder(); for (byte b : hash) { String hex = Integer.toHexString(0xff & b); if (hex.length() == 1) hexString.append('0'); hexString.append(hex); } return hexString.toString(); } }
时间: 2023-07-15 15:11:01 浏览: 45
这段代码实现了一个名为 AdminServiceImpl 的类,它实现了 AdminService 接口。该类使用了 Spring 框架的注解进行依赖注入。其中 @Autowired 注解注入了 AdminDao、DocDao、LogDao、UserDao 和 RoleDao 对象。该类还实现了 login() 方法和 index() 方法,分别用于管理员登录和获取首页数据。其中 login() 方法调用了 AdminDao 的 login() 方法进行管理员身份验证,而 index() 方法则通过查询 DocDao、LogDao、UserDao 和 RoleDao 获得了首页需要显示的各种数据。此外,该类还定义了一个静态的 md5() 方法,用于对密码进行 MD5 加密。
相关问题
@Service @Transactional public class ChatServiceImpl extends AbstractService<Chat> implements ChatService {
这段代码看起来是一个 Java 类的定义,使用了 Spring 的 `@Service` 注解和 Spring 的声明式事务机制 `@Transactional`。该类实现了 `ChatService` 接口,并继承了 `AbstractService<Chat>` 类。根据命名,该类似乎是一个聊天服务实现类。`@Service` 注解表示该类是一个 Spring 的服务类,可以被注入到其他类中使用。`@Transactional` 注解表示该类中的所有方法都将被包装在一个事务中执行,确保数据一致性和事务的原子性。
@service public class UserServicelmpl implements UserService{ @Autowired private UserRepository userRepository; @Override public User findById(Integer id){ return userRepository.fingById(id); } }
在这段代码中,您需要确保您的类上方已经正确地导入了@Service注解,以便您可以使用它。如果您的代码中已经导入了这个注解并且仍然收到“Cannot resolve symbol 'service'”错误,则可能是因为您的项目缺少相应的依赖项。请检查您的项目的依赖项,并确保您已经正确地添加了Spring框架的依赖项。如果您仍然无法解决问题,请提供更多上下文和代码示例,以便我可以更好地帮助您。
相关推荐
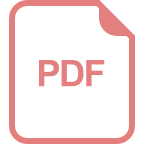
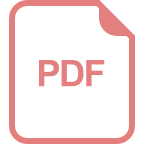
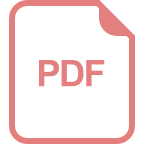













