建立一个单链表,随机产生10个100以内的整数,并按要求完成: (1)在屏幕上显示单链表中的10个整数; (2)删除值为a的结点,若不存在a,则把a插入到第i个位置,显示更新后的单链表;分别用C语言和C++语言完成
时间: 2024-05-31 13:08:11 浏览: 8
C语言代码:
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
struct Node {
int data;
struct Node *next;
};
struct Node *createList(int n) {
struct Node *head, *p, *q;
head = (struct Node *)malloc(sizeof(struct Node));
head->next = NULL;
q = head;
srand(time(0));
for (int i = 0; i < n; i++) {
p = (struct Node *)malloc(sizeof(struct Node));
p->data = rand() % 100 + 1;
q->next = p;
q = p;
}
q->next = NULL;
return head;
}
void printList(struct Node *head) {
struct Node *p = head->next;
while (p) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
void deleteNode(struct Node *head, int a) {
struct Node *p = head->next, *q = head;
while (p) {
if (p->data == a) {
q->next = p->next;
free(p);
return;
}
q = p;
p = p->next;
}
printf("%d not found, insert it to which position? ", a);
int i;
scanf("%d", &i);
p = (struct Node *)malloc(sizeof(struct Node));
p->data = a;
q = head;
for (int j = 0; j < i - 1; j++) {
q = q->next;
}
p->next = q->next;
q->next = p;
}
int main() {
struct Node *head = createList(10);
printf("Original list: ");
printList(head);
int a;
printf("Input a number to delete: ");
scanf("%d", &a);
deleteNode(head, a);
printf("After deletion/insertion: ");
printList(head);
return 0;
}
C++语言代码:
#include <iostream>
#include <cstdlib>
#include <ctime>
using namespace std;
struct Node {
int data;
Node *next;
};
Node *createList(int n) {
Node *head, *p, *q;
head = new Node;
head->next = NULL;
q = head;
srand(time(0));
for (int i = 0; i < n; i++) {
p = new Node;
p->data = rand() % 100 + 1;
q->next = p;
q = p;
}
q->next = NULL;
return head;
}
void printList(Node *head) {
Node *p = head->next;
while (p) {
cout << p->data << " ";
p = p->next;
}
cout << endl;
}
void deleteNode(Node *head, int a) {
Node *p = head->next, *q = head;
while (p) {
if (p->data == a) {
q->next = p->next;
delete p;
return;
}
q = p;
p = p->next;
}
cout << a << " not found, insert it to which position? ";
int i;
cin >> i;
p = new Node;
p->data = a;
q = head;
for (int j = 0; j < i - 1; j++) {
q = q->next;
}
p->next = q->next;
q->next = p;
}
int main() {
Node *head = createList(10);
cout << "Original list: ";
printList(head);
int a;
cout << "Input a number to delete: ";
cin >> a;
deleteNode(head, a);
cout << "After deletion/insertion: ";
printList(head);
return 0;
}
相关推荐
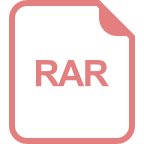














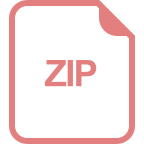