基于c#的简易逆波兰式算法的计算器,包含平方、开方、三角函数、log、加减乘除,写出窗体程序代码,以及各控件属性。
时间: 2023-07-10 21:35:28 浏览: 154
以下是基于C#编写的简易逆波兰式计算器窗体程序。该程序支持平方、开方、三角函数、log、加减乘除等操作。
在窗体中添加以下控件:
- 一个Label控件用于显示当前表达式。
- 一个TextBox控件用于接收用户输入的表达式。
- 一个Button控件,点击该按钮后将会计算表达式并显示结果。
- 一个ComboBox控件,用于选择需要执行的操作,比如平方、开方、三角函数等。
- 一个ListBox控件,用于显示计算结果。
以下是该窗体程序的代码:
```csharp
using System;
using System.Collections.Generic;
using System.Windows.Forms;
namespace Calculator
{
public partial class Form1 : Form
{
// Create a stack to store operands
private Stack<double> stack = new Stack<double>();
public Form1()
{
InitializeComponent();
}
private void buttonCalculate_Click(object sender, EventArgs e)
{
// Get the input expression from the textbox
string input = textBoxExpression.Text;
// Append the selected operation to the input expression
string operation = comboBoxOperation.Text;
if (!string.IsNullOrEmpty(operation))
{
input += " " + operation;
}
// Convert the input expression to a list of tokens
List<string> tokens = new List<string>(input.Split());
// Clear the stack
stack.Clear();
// Evaluate the expression
foreach (string token in tokens)
{
double operand;
if (double.TryParse(token, out operand))
{
// If the token is a number, push it onto the stack
stack.Push(operand);
}
else
{
// If the token is an operator or function, pop one or two operands from the stack and apply the operator or function
double operand2 = 0;
double operand1 = 0;
double result = 0;
switch (token)
{
case "+":
operand2 = stack.Pop();
operand1 = stack.Pop();
result = operand1 + operand2;
break;
case "-":
operand2 = stack.Pop();
operand1 = stack.Pop();
result = operand1 - operand2;
break;
case "*":
operand2 = stack.Pop();
operand1 = stack.Pop();
result = operand1 * operand2;
break;
case "/":
operand2 = stack.Pop();
operand1 = stack.Pop();
result = operand1 / operand2;
break;
case "^":
operand2 = stack.Pop();
operand1 = stack.Pop();
result = Math.Pow(operand1, operand2);
break;
case "sqrt":
operand1 = stack.Pop();
result = Math.Sqrt(operand1);
break;
case "sin":
operand1 = stack.Pop();
result = Math.Sin(operand1);
break;
case "cos":
operand1 = stack.Pop();
result = Math.Cos(operand1);
break;
case "tan":
operand1 = stack.Pop();
result = Math.Tan(operand1);
break;
case "log":
operand1 = stack.Pop();
result = Math.Log10(operand1);
break;
default:
MessageBox.Show("Invalid operator or function: " + token);
return;
}
// Push the result back onto the stack
stack.Push(result);
}
}
// The final result is on the top of the stack
double finalResult = stack.Pop();
// Display the result in the listbox
listBoxResults.Items.Add(input + " = " + finalResult);
// Clear the input textbox
textBoxExpression.Clear();
// Set the focus back to the textbox
textBoxExpression.Focus();
}
}
}
```
在窗体设计器中,需要对各控件进行如下属性设置:
- Label控件:AutoSize = true, Text = “Expression:”
- TextBox控件:Dock = Top, Multiline = true, Height = 100
- Button控件:Dock = Top, Text = “Calculate”, Height = 30
- ComboBox控件:Dock = Top, Items = “”, “sqrt”, “sin”, “cos”, “tan”, “log”, “^”, Text = “”
- ListBox控件:Dock = Fill
这样就可以实现一个基于C#的简易逆波兰式计算器窗体程序了。
阅读全文
相关推荐
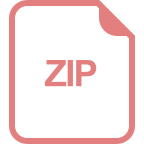
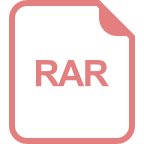
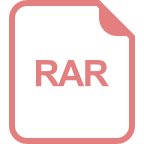
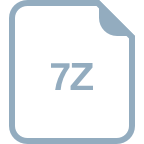
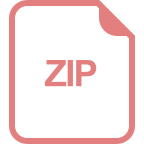
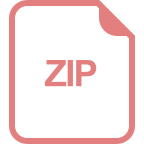
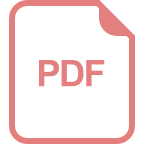
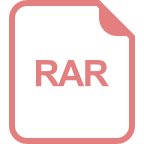
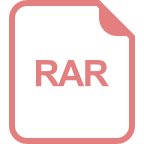
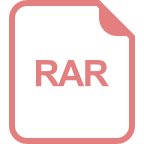
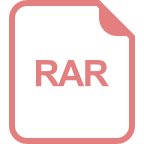
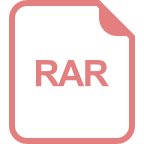
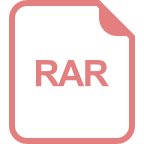
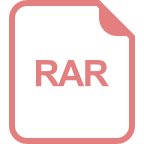
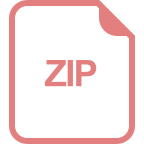
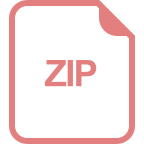
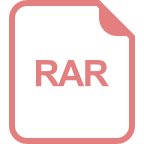