在微信小程序JS中自定义获取当前日期函数,传递给前端
时间: 2024-03-25 19:35:47 浏览: 24
在微信小程序JS中自定义获取当前日期的函数,可以通过以下方式实现:
```
function getCurrentDate() {
// 获取当前日期
const today = new Date();
const year = today.getFullYear();
const month = today.getMonth() + 1;
const day = today.getDate();
// 返回日期字符串
return year + '-' + month + '-' + day;
}
Page({
data: {
currentDate: ''
},
onLoad: function () {
// 调用自定义函数获取当前日期
const currentDate = getCurrentDate();
// 将日期传递给前端
this.setData({
currentDate: currentDate
});
}
})
```
在这个例子中,我们定义了一个名为getCurrentDate的函数,用来获取当前日期,并返回日期字符串。然后,在页面加载时,我们调用该函数获取当前日期,并将其传递给前端。在前端页面中,我们可以使用{{currentDate}}来显示当前日期。
相关问题
在微信小程序JS中自定义获取当前日期函数,并将自定义参数传递给前端
在微信小程序JS中自定义获取当前日期函数,并将自定义参数传递给前端,可以通过以下方式实现:
```
function getCurrentDate(format) {
// 获取当前日期
const today = new Date();
const year = today.getFullYear();
const month = today.getMonth() + 1;
const day = today.getDate();
// 根据传入的格式化参数返回日期字符串
if (format === 'yyyy-mm-dd') {
return year + '-' + month + '-' + day;
} else if (format === 'mm/dd/yyyy') {
return month + '/' + day + '/' + year;
} else {
return year + '/' + month + '/' + day;
}
}
Page({
data: {
currentDate: ''
},
onLoad: function () {
// 调用自定义函数获取当前日期
const currentDate = getCurrentDate('yyyy-mm-dd');
// 将日期传递给前端
this.setData({
currentDate: currentDate
});
}
})
```
在这个例子中,我们定义了一个名为getCurrentDate的函数,用来获取当前日期,并根据传入的format参数返回不同格式的日期字符串。在页面加载时,我们调用该函数获取当前日期,并将其传递给前端。在这个例子中,我们传入了'yyyy-mm-dd'作为format参数,因此返回的日期字符串格式为'年-月-日'。在前端页面中,我们可以使用{{currentDate}}来显示当前日期。
在微信小程序JS中获取当前日期,传递给前端
在微信小程序JS中,可以使用JavaScript内置的Date对象来获取当前日期。可以通过以下代码获取当前日期,并将其传递给前端:
```
Page({
data: {
currentDate: ''
},
onLoad: function () {
// 获取当前日期
const today = new Date();
const year = today.getFullYear();
const month = today.getMonth() + 1;
const day = today.getDate();
// 将日期传递给前端
this.setData({
currentDate: year + '-' + month + '-' + day
});
}
})
```
在这个例子中,我们在页面加载时获取了当前日期,并将其赋值给data中的currentDate属性。然后,我们可以在前端页面中使用{{currentDate}}来显示当前日期。
相关推荐
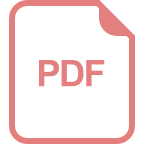
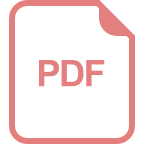
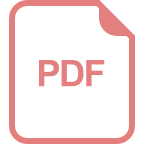












