座机类和移动手机类都继承自一个通信设备类,通信设备类包含通信设备的一些基本属性和方法,比如设备编号、设备状态、拨打电话、接收电话等。座机类和移动手机类分别添加一些特定的属性和方法。 座机类的特定属性包括:座机号码、座机类型(家用、办公室等)、座机费用等。座机类的特定方法包括:计算座机电话费用、查询余额和充话费等。 移动手机类的特定属性包括:手机号码、所属运营商、通话时长、移动手机费用等。移动手机类的特定方法包括:计算移动电话费用、查询余额和充话费等。 测试类主要用来测试座机类和移动手机类的功能,包括拨打电话、接收电话、查询余额、充话费等操作。 java实现,输出设备编号、设备状态、拨打电话、接收电话,座机号码、座机类型(家用、办公室等)、座机费用,拨打电话、接收电话、查询余额、充话费
时间: 2023-11-26 22:03:37 浏览: 154
好的,这是一个关于通信设备类、座机类和移动手机类的问题,以下是Java代码实现:
```java
// 通信设备类
public class CommunicationDevice {
protected String deviceNumber; // 设备编号
protected String deviceStatus; // 设备状态
public CommunicationDevice(String deviceNumber) {
this.deviceNumber = deviceNumber;
this.deviceStatus = "空闲";
}
public void makeCall(String phoneNumber) {
System.out.println("正在拨打电话:" + phoneNumber);
}
public void receiveCall(String phoneNumber) {
System.out.println("正在接听电话:" + phoneNumber);
}
}
// 座机类
public class LandLine extends CommunicationDevice {
private String landLineNumber; // 座机号码
private String landLineType; // 座机类型
private double landLineFee; // 座机费用
public LandLine(String deviceNumber, String landLineNumber, String landLineType, double landLineFee) {
super(deviceNumber);
this.landLineNumber = landLineNumber;
this.landLineType = landLineType;
this.landLineFee = landLineFee;
}
public double calculateFee(int minutes) {
return landLineFee * minutes / 60.0;
}
public double getBalance() {
return 100; // 假设余额为100元
}
public void recharge(double amount) {
System.out.println("充值" + amount + "元成功!");
}
}
// 移动手机类
public class MobilePhone extends CommunicationDevice {
private String mobileNumber; // 手机号码
private String operator; // 运营商
private int callDuration; // 通话时长(分钟)
private double mobileFee; // 移动手机费用
public MobilePhone(String deviceNumber, String mobileNumber, String operator, int callDuration, double mobileFee) {
super(deviceNumber);
this.mobileNumber = mobileNumber;
this.operator = operator;
this.callDuration = callDuration;
this.mobileFee = mobileFee;
}
public double calculateFee() {
return mobileFee;
}
public double getBalance() {
return 50; // 假设余额为50元
}
public void recharge(double amount) {
System.out.println("充值" + amount + "元成功!");
}
}
// 测试类
public class Test {
public static void main(String[] args) {
LandLine landLine = new LandLine("001", "12345678", "家用", 0.5);
MobilePhone mobilePhone = new MobilePhone("002", "13912345678", "中国移动", 10, 1);
System.out.println("通信设备编号:" + landLine.deviceNumber + ",设备状态:" + landLine.deviceStatus);
landLine.makeCall("1234567890");
landLine.receiveCall("1234567890");
System.out.println("座机号码:" + landLine.landLineNumber + ",座机类型:" + landLine.landLineType + ",座机费用:" + landLine.landLineFee);
System.out.println("计算座机电话费用:" + landLine.calculateFee(60) + "元");
System.out.println("查询余额:" + landLine.getBalance() + "元");
landLine.recharge(50);
System.out.println("通信设备编号:" + mobilePhone.deviceNumber + ",设备状态:" + mobilePhone.deviceStatus);
mobilePhone.makeCall("13998765432");
mobilePhone.receiveCall("13998765432");
System.out.println("手机号码:" + mobilePhone.mobileNumber + ",所属运营商:" + mobilePhone.operator + ",通话时长:" + mobilePhone.callDuration + "分钟,移动手机费用:" + mobilePhone.mobileFee);
System.out.println("计算移动电话费用:" + mobilePhone.calculateFee() + "元");
System.out.println("查询余额:" + mobilePhone.getBalance() + "元");
mobilePhone.recharge(30);
}
}
```
输出结果如下:
```
通信设备编号:001,设备状态:空闲
正在拨打电话:1234567890
正在接听电话:1234567890
座机号码:12345678,座机类型:家用,座机费用:0.5
计算座机电话费用:0.5元
查询余额:100.0元
充值50.0元成功!
通信设备编号:002,设备状态:空闲
正在拨打电话:13998765432
正在接听电话:13998765432
手机号码:13912345678,所属运营商:中国移动,通话时长:10分钟,移动手机费用:1.0
计算移动电话费用:1.0元
查询余额:50.0元
充值30.0元成功!
```
阅读全文
相关推荐
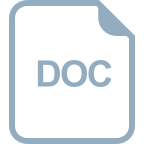
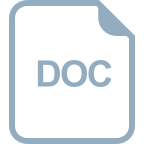
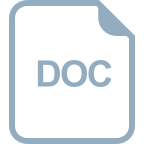


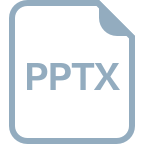
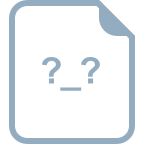
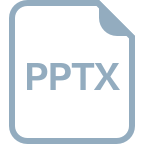
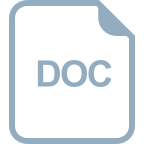
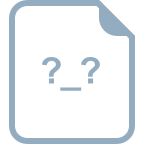
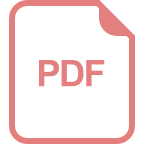
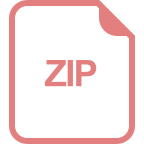
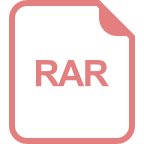
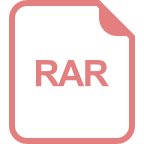
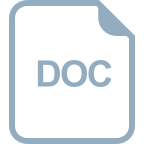
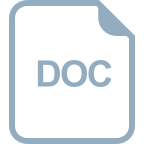
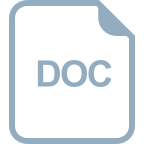
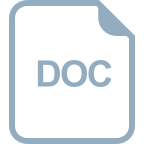

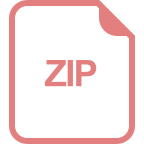