2设计一个银行账户类,该类具有以下属性和方法:账户号码、账户类型、余额、存款、取款。其中存款和取款方法会改变余额
时间: 2023-07-10 08:21:28 浏览: 209
好的,以下是一个简单的银行账户类的代码示例,实现了所述的属性和方法:
```python
class BankAccount:
def __init__(self, account_number, account_type, balance):
self.account_number = account_number
self.account_type = account_type
self.balance = balance
def deposit(self, amount):
self.balance += amount
def withdraw(self, amount):
if self.balance >= amount:
self.balance -= amount
else:
print("Insufficient balance")
def __str__(self):
return f"Account number: {self.account_number}, Account type: {self.account_type}, Balance: {self.balance}"
```
在这个类中,`__init__`方法用于初始化账户号码、账户类型和余额属性,`deposit`方法用于向账户中存入指定金额,`withdraw`方法用于从账户中取出指定金额,`__str__`方法用于返回一个字符串,包含账户号码、账户类型和余额信息。注意,`withdraw`方法会检查余额是否足够,如果不足,会输出一条“余额不足”的消息。
可以使用以下代码创建一个银行账户对象并进行测试:
```python
account = BankAccount("123456789", "Savings", 1000)
print(account)
# Output: Account number: 123456789, Account type: Savings, Balance: 1000
account.deposit(500)
print(account)
# Output: Account number: 123456789, Account type: Savings, Balance: 1500
account.withdraw(200)
print(account)
# Output: Account number: 123456789, Account type: Savings, Balance: 1300
account.withdraw(2000)
# Output: Insufficient balance
print(account)
# Output: Account number: 123456789, Account type: Savings, Balance: 1300
```
在这个例子中,我们首先创建了一个银行账户对象,账户号码为“123456789”,账户类型为“Savings”,余额为1000。然后,我们使用`deposit`方法存入了500元,使用`withdraw`方法取出了200元。最后,我们试图从账户中取出2000元,但是因为余额不足,输出了一条“余额不足”的消息。
阅读全文
相关推荐

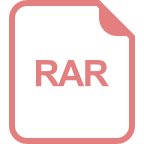














