用c++写一段程序, 输入一个字符,判断是不是字母,小写的话输出其大写形式,大写的话输出其小写形式,否则显示不是字母
时间: 2024-05-12 21:19:41 浏览: 54
```c
#include <stdio.h>
int main() {
char c;
printf("请输入一个字符:");
scanf("%c", &c);
if (c >= 'a' && c <= 'z') {
printf("%c\n", c - 'a' + 'A');
} else if (c >= 'A' && c <= 'Z') {
printf("%c\n", c - 'A' + 'a');
} else {
printf("不是字母\n");
}
return 0;
}
```
相关问题
用c++写一段程序,判断是不是字母小写的话输出大写形式大写的话输出小写形式否则显示不是字母
```c
#include <stdio.h>
int main() {
char c;
printf("请输入一个字符:");
scanf("%c", &c);
if (c >= 'a' && c <= 'z') {
printf("大写形式:%c\n", c - 'a' + 'A');
} else if (c >= 'A' && c <= 'Z') {
printf("小写形式:%c\n", c - 'A' + 'a');
} else {
printf("不是字母!\n");
}
return 0;
}
```
c++输入一个小写字母输出其对应大写字母不使用条件语句
在 C++ 中,如果你想要将小写字母转换成大写字母,而又不想使用条件语句(如 `if`、`switch` 等),你可以利用 C++ 标准库提供的函数 `toupper()`,它属于 `<cctype>` 头文件的一部分。这个函数接受一个字符作为输入,并返回对应的ASCII码值的大写版本。
以下是一个简单的示例,展示了如何通过位操作实现这一功能:
```cpp
#include <iostream>
#include <cctype>
char toUpperCase(char c) {
// ASCII码表中小写a到z的值在97到122之间
if (c >= 'a' && c <= 'z') {
// 使用位操作,将第五位(从左数起)设置为1,变成大写
return static_cast<char>(c | 0x20);
} else {
// 如果不是小写字母则不变
return c;
}
}
int main() {
char lowerCaseChar;
std::cin >> lowerCaseChar;
std::cout << toUpperCase(lowerCaseChar) << std::endl;
return 0;
}
```
在这个例子中,我们假设输入的是小写字母,并通过位运算将其第五位(ASCII码表中的“大写”标志)置1,从而实现转换。如果不是小写字母,则直接返回原样。
阅读全文
相关推荐
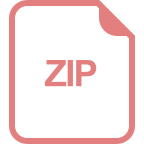
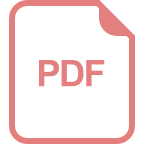













