antd中点击Form.Item的label触发了Switch改变
时间: 2024-03-05 19:48:24 浏览: 194
我了解您的问题。您可以通过在 `<Form.Item>` 中添加 `htmlFor` 属性并将其设置为 `Switch` 组件的 `id` 值来实现这一功能。例如:
```
<Form.Item label="开关" htmlFor="switch">
<Switch id="switch" onChange={handleChange} />
</Form.Item>
```
这样,当您单击标签时,它将自动触发关联的 `Switch` 组件的更改事件。
相关问题
react antd-design Form.Item 的label属性上加 Tooltip复制功能,复制内容为input输入框中的内容 点击Tooltip提示·即可直接复制
您好!根据您的需求,我可以给出以下解决思路:
1. 在需要添加 Tooltip 复制功能的 Form.Item 的 label 属性上添加 Tooltip 组件,并设置其 title 属性为需要复制的内容;
2. 在 Tooltip 组件中添加 onClick 事件,并在该事件中获取 input 输入框的 value 值,并将其复制到剪贴板中实现复制功能。
下面是具体的代码实现,仅供参考:
```
import React from 'react';
import { Form, Input, Tooltip } from 'antd';
import { CopyOutlined } from '@ant-design/icons';
const Demo = () => {
const handleCopy = () => {
const { value } = inputRef.current.input;
const textarea = document.createElement('textarea');
textarea.innerText = value;
document.body.appendChild(textarea);
textarea.select();
document.execCommand('copy');
document.body.removeChild(textarea);
};
const inputRef = React.useRef(null);
return (
<Form>
<Form.Item
label={
<Tooltip
title={
<div onClick={handleCopy}>
点击复制:
<br />
{inputRef.current?.input?.value}
</div>
}
placement="topLeft"
>
需要复制的内容:
</Tooltip>
}
>
<Input ref={inputRef} />
</Form.Item>
</Form>
);
};
export default Demo;
```
在上述代码中,我们在 Tooltip 组件的 title 属性中添加了一个可点击的 div 元素,并给该元素添加了 onClick 事件,该事件中获取 input 输入框的 value 值,并将其复制到剪贴板中。通过这种方式,当用户点击 Tooltip 组件时,即可直接复制内容到剪贴板中。
希望这可以帮助到您!如果您还有其他问题,可以继续提问。
antd Form.List中的一个Form.Item如何获取其他Form.Item的值
在 antd Form.List 中,一个 Form.Item 可以通过使用 getFieldDecorator 方法来获取自身的值,而获取其他 Form.Item 的值则需要通过 Form.List 的方法进行。例如,可以通过调用 form.getFieldValue 方法来获取其他 Form.Item 的值。具体实现步骤如下:
1. 在 Form.List 中,给每个 Form.Item 一个唯一的 name 属性,例如 name={`items[${index}].name`},其中 index 为当前 Form.Item 的索引。
2. 在 getFieldDecorator 方法中,使用 getFieldValue 方法来获取其他 Form.Item 的值。示例代码如下:
```jsx
{getFieldDecorator(`items[${index}].name`, {
initialValue: item.name,
rules: [{ required: true, message: 'Please input name' }],
})(
<Input placeholder="Enter name" />
)}
{getFieldDecorator(`items[${index}].age`, {
initialValue: item.age,
rules: [{ required: true, message: 'Please input age' }],
})(
<InputNumber placeholder="Enter age" min={0} />
)}
{getFieldDecorator(`items[${index}].email`, {
initialValue: item.email,
rules: [{ required: true, message: 'Please input email' }],
})(
<Input placeholder="Enter email" />
)}
```
3. 在需要获取其他 Form.Item 值的地方,调用 form.getFieldValue 方法。示例代码如下:
```jsx
const handleSubmit = e => {
e.preventDefault();
form.validateFields((err, values) => {
if (!err) {
// 获取其他 Form.Item 值
const names = form.getFieldValue('items').map(item => item.name);
console.log(names);
// 提交表单
console.log('Received values of form: ', values);
}
});
};
```
阅读全文
相关推荐
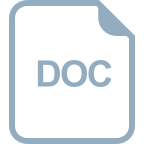
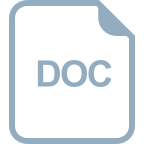
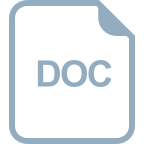
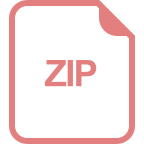
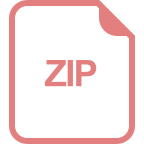











