在antd中当form设置layout为horizontal时,如何将单个Form.Item中的元素设置为vertical
时间: 2024-03-27 18:40:36 浏览: 174
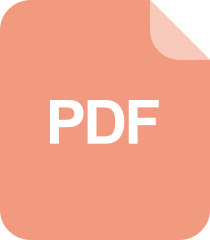
antd Form组件行并列显示 Form表单属性为inline时,表单组件宽度问题

可以使用 `flex` 属性实现。在需要设置为 `vertical` 的 `Form.Item` 中添加一个 `div`,并设置其样式为 `display: flex; flex-direction: column;`,然后将需要垂直排列的元素放到这个 `div` 中即可。
示例代码如下:
```jsx
<Form layout="horizontal">
<Form.Item label="Label">
<div style={{ display: 'flex', flexDirection: 'column' }}>
<Input placeholder="Input" />
<Button>Button</Button>
</div>
</Form.Item>
<Form.Item label="Label">
<Input placeholder="Input" />
</Form.Item>
</Form>
```
阅读全文
相关推荐
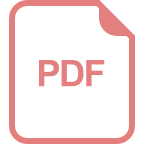
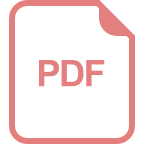

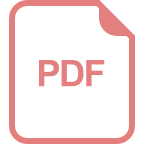
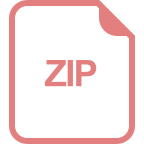












