react 腾讯对象存储js直传方法
时间: 2023-10-09 20:05:33 浏览: 36
使用腾讯云对象存储(COS)的 JavaScript 直传方法需要安装 COS SDK for JavaScript 和 React。
以下是一个基本的 React 组件,可以将文件直接上传到 COS:
```
import React, { useState } from 'react';
import COS from 'cos-js-sdk-v5';
const Bucket = 'your-bucket-name';
const Region = 'your-region';
const SecretId = 'your-secret-id';
const SecretKey = 'your-secret-key';
function App() {
const [file, setFile] = useState(null);
const [progress, setProgress] = useState(0);
const handleFileChange = (event) => {
setFile(event.target.files[0]);
};
const handleUpload = () => {
if (!file) return;
const cos = new COS({
SecretId,
SecretKey,
});
const key = file.name;
cos.putObject(
{
Bucket,
Region,
Key: key,
Body: file,
onProgress: function (progressData) {
setProgress(progressData.percent * 100);
},
},
(err, data) => {
if (err) {
console.error(err);
} else {
console.log(data);
}
}
);
};
return (
<div>
<input type="file" onChange={handleFileChange} />
<button onClick={handleUpload}>Upload</button>
{file && <p>Selected file: {file.name}</p>}
{progress > 0 && <p>Progress: {progress}%</p>}
</div>
);
}
export default App;
```
在上面的代码中,我们使用 `cos-js-sdk-v5` 包来创建一个 COS 对象并上传文件。在 `onProgress` 函数中,我们可以获取上传进度并在 UI 中显示它。
请注意,您需要将 `Bucket`、`Region`、`SecretId` 和 `SecretKey` 替换为您的实际值。
相关推荐
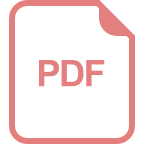
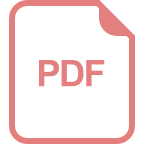














