卷积神经网络求信噪比代码实例
时间: 2024-03-15 11:40:49 浏览: 31
当使用卷积神经网络(Convolutional Neural Network,CNN)进行图像处理时,可以通过计算信噪比(Signal-to-Noise Ratio,SNR)来评估图像的质量。下面是一个使用Python实现的卷积神经网络求信噪比的代码示例:
```python
import numpy as np
import tensorflow as tf
# 构建卷积神经网络模型
def build_model():
model = tf.keras.Sequential([
tf.keras.layers.Conv2D(32, (3, 3), activation='relu', input_shape=(28, 28, 1)),
tf.keras.layers.MaxPooling2D((2, 2)),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(64, activation='relu'),
tf.keras.layers.Dense(10, activation='softmax')
])
return model
# 计算信噪比
def calculate_snr(original_image, noisy_image):
mse = np.mean((original_image - noisy_image) ** 2)
if mse == 0:
return 100
max_pixel = np.max(original_image)
snr = 10 * np.log10(max_pixel ** 2 / mse)
return snr
# 加载数据集
(x_train, y_train), (x_test, y_test) = tf.keras.datasets.mnist.load_data()
# 数据预处理
x_train = x_train.reshape(-1, 28, 28, 1) / 255.0
x_test = x_test.reshape(-1, 28, 28, 1) / 255.0
# 构建并训练模型
model = build_model()
model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy'])
model.fit(x_train, y_train, epochs=5)
# 生成噪声图像
noisy_images = x_test + np.random.normal(loc=0, scale=0.1, size=x_test.shape)
# 预测图像
predicted_images = model.predict(noisy_images)
# 计算信噪比
snr_values = []
for i in range(len(x_test)):
snr = calculate_snr(x_test[i], predicted_images[i])
snr_values.append(snr)
# 打印信噪比结果
print("SNR values:", snr_values)
```
上述代码中,首先构建了一个简单的卷积神经网络模型,然后使用MNIST数据集进行训练。接着,生成了一组噪声图像,并使用训练好的模型对这些噪声图像进行预测。最后,通过计算原始图像和预测图像之间的均方误差(Mean Squared Error,MSE)来计算信噪比。
请注意,上述代码仅为示例,实际应用中可能需要根据具体情况进行修改和调整。
相关推荐
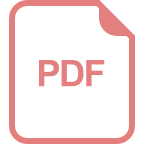
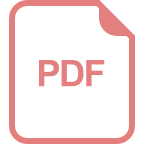
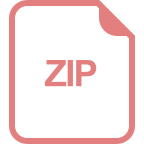














