3. 编写一个函数 text_three(arr),接收一个由整数组成的列表 arr,找到该列表中连续子数组的最大乘积。子数组必须至少包含一个元素
时间: 2024-09-19 17:16:37 浏览: 39
text_three 函数的主要目的是找到给定整数列表 arr 中具有最大乘积的连续子数组。这个任务通常通过动态规划或者分治法来解决,因为可以考虑维护两个变量:当前乘积(current_product)和到目前为止遇到的最大乘积(max_product)。算法会遍历数组,对于每个元素:
1. 如果当前乘积是正数,直接将其与当前元素相乘,更新当前乘积。
2. 如果当前乘积为负,表示之前的序列乘积是负的,这时应该从当前元素开始一个新的序列,因此将当前元素设置为新的当前乘积。
3. 每次遍历结束后,如果当前乘积大于已知的最大乘积,就更新最大乘积。
这是一个伪代码示例:
```python
def text_three(arr):
if not arr:
return 0
current_product = max_product = arr[0]
for i in range(1, len(arr)):
current_product = max(arr[i], current_product * arr[i]) # 取较大值,要么保持乘积要么清零
max_product = max(max_product, current_product)
return max_product
```
相关问题
编写一个函数 text three(arr),接收一个由整数组成的列表 ar,找到该列表中连续子数组的最大乘积。子数组必须至少包含一个元素。
在编写一个名为 `text_three` 的函数,用于计算给定整数数组 `arr` 中连续子数组的最大乘积时,可以采用动态规划的方法。首先初始化两个变量,`max_product` 和 `current_product`,分别记录当前最大乘积和当前连续乘积。遍历数组,对于每个元素,有三种情况:
1. 如果当前元素大于0,直接将 `current_product` 加上该元素,因为正数相乘结果肯定是正数。
2. 如果当前元素小于等于0,有两种可能:要么保留当前元素,开始一个新的序列;要么更新 `current_product` 为该元素本身,因为乘以0或负数会使得乘积变小。
3. 比较 `current_product` 与 `max_product`,如果 `current_product` 更大,则更新 `max_product`。
函数的伪代码如下:
```python
def text_three(arr):
max_product = arr[0] if arr else 0
current_product = max_product
for i in range(1, len(arr)):
if arr[i] > 0:
current_product *= arr[i]
elif arr[i] <= 0:
current_product = max(arr[i], current_product * arr[i])
max_product = max(max_product, current_product)
return max_product
```
1.编写重载函数max1可分别求取2个整数、3个整数、2个双精度数、3个双精度数的最大值。 2.用new操作为一个包含10个整数的数组分配内存,输入若干个值到数组中,分别统计其中正数和负数的个数后再用delete操作释放内存。(c++)
1. 在C++中,你可以通过重载函数来实现`max1`的不同版本,以便处理不同类型的参数。这里是一个例子:
```cpp
#include <iostream>
using namespace std;
// 版本一:2个整数
int max1(int a, int b) {
return (a > b) ? a : b;
}
// 版本二:3个整数
int max1(int a, int b, int c) {
return max(max(a, b), c);
}
// 版本三:2个double
double max1(double a, double b) {
return (a > b) ? a : b;
}
// 版本四:3个double
double max1(double a, double b, double c) {
return max(max(a, b), c);
}
// 调用示例:
int main() {
cout << "Max of two integers: " << max1(5, 10) << endl;
cout << "Max of three integers: " << max1(1, 2, 3) << endl;
cout << "Max of two doubles: " << max1(1.5, 2.7) << endl;
// 更多版本可以类似地添加...
return 0;
}
```
2. 对于动态数组,首先需要定义结构体并分配内存,然后读取输入并统计正负数:
```cpp
#include <iostream>
using namespace std;
struct ArrayStats {
int positiveCount;
int negativeCount;
};
void processArray(int* arr, int size) {
ArrayStats stats = {0, 0};
for (int i = 0; i < size; i++) {
if (arr[i] > 0) {
stats.positiveCount++;
} else if (arr[i] < 0) {
stats.negativeCount++;
}
}
cout << "Positive numbers: " << stats.positiveCount << endl;
cout << "Negative numbers: " << stats.negativeCount << endl;
}
int main() {
int arraySize = 10;
int* myArray = new int[arraySize];
// 读取用户输入并存储到数组
for (int i = 0; i < arraySize; i++) {
cin >> myArray[i];
}
processArray(myArray, arraySize);
delete[] myArray; // 释放内存
return 0;
}
```
阅读全文
相关推荐
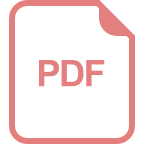
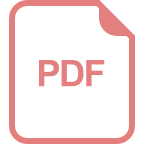
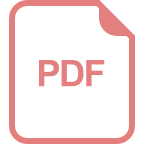
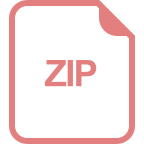
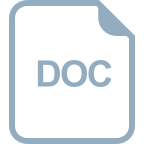
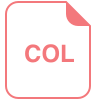
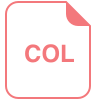
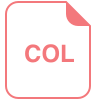
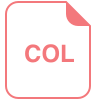







