priority_queue存struct
时间: 2023-11-06 17:09:09 浏览: 101
可以使用priority_queue存储struct,只需要在定义priority_queue时指定比较函数即可。比较函数需要返回bool类型,表示两个元素的优先级大小关系。
例如,定义一个结构体Person,包含姓名和年龄两个成员变量,按照年龄从小到大排序:
```cpp
struct Person {
string name;
int age;
};
struct cmp {
bool operator() (const Person& a, const Person& b) {
return a.age > b.age; // 按照年龄从小到大排序
}
};
priority_queue<Person, vector<Person>, cmp> pq;
```
相关问题
priority_queue以struct为存贮的使用方式
priority_queue以struct为存储的使用方式如下所示:
```cpp
#include <iostream>
#include <queue>
using namespace std;
struct Student {
string name;
int score;
};
struct Compare {
bool operator()(const Student& s1, const Student& s2) {
return s1.score < s2.score; // 按照分数从小到大排序
}
};
int main() {
priority_queue<Student, vector<Student>, Compare> pq;
Student s1 = {"Alice", 85};
Student s2 = {"Bob", 92};
Student s3 = {"Charlie", 78};
pq.push(s1);
pq.push(s2);
pq.push(s3);
while (!pq.empty()) {
Student top = pq.top();
pq.pop();
cout << "Name: " << top.name << ", Score: " << top.score << endl;
}
return 0;
}
```
在上述代码中,我们定义了一个`Student`结构体,包含学生的姓名和分数。然后,我们定义了一个`Compare`结构体,重载了`()`运算符,用于比较两个学生的分数大小。接下来,在主函数中,我们创建了一个`priority_queue`,指定存储的元素类型为`Student`,底层容器为`vector`,比较函数为`Compare`。然后,我们创建了三个学生对象,并将它们依次插入到优先级队列中。最后,我们通过循环取出队列中的元素,并输出学生的姓名和分数。
struct priority_queue
这是一个 C++ 中的优先队列数据结构,它可以自动维护队列中元素的优先级,使得每次取出的元素都是当前队列中优先级最高的元素。
优先队列的实现方式有多种,常见的有基于堆的实现和基于红黑树的实现。在 C++ STL 中,优先队列默认使用基于堆的实现。
优先队列支持插入元素、删除队首元素、获取队首元素等操作,时间复杂度为 O(log n)。
阅读全文
相关推荐
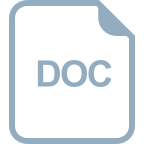
















