用c语言,实现函数 void uint32_encode(uint32_t value, uint8_t* p_encoded_data)4//把 value 按照小端序的方式存放 p_encoded data 数组里
时间: 2023-08-05 20:41:22 浏览: 295
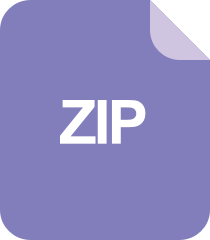
code uint8-t *p-flash 与uint8-t code *p-flash的区别
以下是使用c语言实现函数 `void uint32_encode(uint32_t value, uint8_t* p_encoded_data)` 的代码:
```c
void uint32_encode(uint32_t value, uint8_t* p_encoded_data) {
p_encoded_data[0] = (uint8_t)value;
p_encoded_data[1] = (uint8_t)(value >> 8);
p_encoded_data[2] = (uint8_t)(value >> 16);
p_encoded_data[3] = (uint8_t)(value >> 24);
}
```
该函数将一个32位的无符号整数按照小端序的方式存放在一个长度为4的字节数组中。函数的第一个参数为要编码的整数值,第二个参数为存放编码结果的字节数组。
实现思路是:先将整数的最低8位存放在字节数组的第一个元素中,然后依次将整数的高8位存放在字节数组中的后续元素中,直到整个32位整数都被存放在了字节数组中。
阅读全文
相关推荐
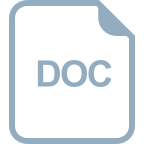
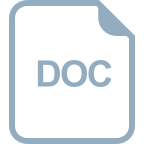

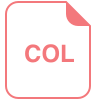
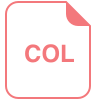
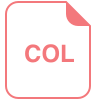











