egg+typescript+mysql
时间: 2023-06-28 17:05:02 浏览: 60
Egg.js 是一个基于 Node.js 和 Koa 的 Web 框架,它提供了很多便捷的功能和插件,让开发者可以快速地构建 Web 应用程序。TypeScript 则是一种静态类型检查的编程语言,可以帮助我们在编写代码时更早地捕获一些错误,提高代码的可维护性和可读性。MySQL 则是一种常用的关系型数据库,用于存储和管理数据。
在 Egg.js 中使用 TypeScript 和 MySQL,我们可以使用 egg-mysql 插件来连接和操作 MySQL 数据库,并使用 TypeScript 的类型检查来保证代码的正确性。具体步骤如下:
1. 创建 Egg.js 项目
```bash
$ npm i egg-init -g
$ egg-init egg-typescript-mysql --type=ts
$ cd egg-typescript-mysql
$ npm i
```
2. 安装 egg-mysql 插件
```bash
$ npm i egg-mysql
```
3. 配置 MySQL 数据库连接
在 `config/config.default.ts` 中添加以下配置:
```typescript
config.mysql = {
client: {
host: 'localhost',
port: '3306',
user: 'root',
password: 'password',
database: 'test',
},
app: true,
agent: false,
};
```
其中,`host`、`port`、`user`、`password`、`database` 分别为你的 MySQL 数据库连接信息。
4. 创建 Model
在 `app/model` 目录下创建一个新的文件 `user.ts`,用于定义操作用户数据的 Model:
```typescript
import { Application } from 'egg';
export default (app: Application) => {
const { INTEGER, STRING } = app.Sequelize;
const User = app.model.define('user', {
id: {
type: INTEGER,
primaryKey: true,
autoIncrement: true,
},
name: {
type: STRING(50),
allowNull: false,
},
age: {
type: INTEGER,
allowNull: false,
},
});
return User;
};
```
其中,`app.Sequelize` 是通过 egg-sequelize 插件提供的 Sequelize 实例。
5. 创建 Controller 和 Service
在 `app/controller` 目录下创建一个新的文件 `user.ts`,用于处理用户相关的请求:
```typescript
import { Controller } from 'egg';
export default class UserController extends Controller {
async index() {
const { ctx } = this;
const users = await ctx.service.user.list();
ctx.body = { users };
}
async show() {
const { ctx } = this;
const user = await ctx.service.user.get(ctx.params.id);
ctx.body = { user };
}
async create() {
const { ctx } = this;
const { name, age } = ctx.request.body;
const user = await ctx.service.user.create(name, age);
ctx.body = { user };
}
async update() {
const { ctx } = this;
const { id, name, age } = ctx.request.body;
const user = await ctx.service.user.update(id, name, age);
ctx.body = { user };
}
async destroy() {
const { ctx } = this;
const user = await ctx.service.user.delete(ctx.params.id);
ctx.body = { user };
}
}
```
在 `app/service` 目录下创建一个新的文件 `user.ts`,用于实现对用户数据的操作:
```typescript
import { Service } from 'egg';
export default class UserService extends Service {
async list() {
const { ctx } = this;
const users = await ctx.model.User.findAll();
return users;
}
async get(id: number) {
const { ctx } = this;
const user = await ctx.model.User.findByPk(id);
return user;
}
async create(name: string, age: number) {
const { ctx } = this;
const user = await ctx.model.User.create({ name, age });
return user;
}
async update(id: number, name: string, age: number) {
const { ctx } = this;
const user = await ctx.model.User.findByPk(id);
if (!user) {
ctx.throw(404, 'user not found');
}
await user.update({ name, age });
return user;
}
async delete(id: number) {
const { ctx } = this;
const user = await ctx.model.User.findByPk(id);
if (!user) {
ctx.throw(404, 'user not found');
}
await user.destroy();
return user;
}
}
```
6. 路由配置
在 `app/router.ts` 中配置路由:
```typescript
import { Application } from 'egg';
export default (app: Application) => {
const { controller, router } = app;
router.get('/users', controller.user.index);
router.get('/users/:id', controller.user.show);
router.post('/users', controller.user.create);
router.put('/users', controller.user.update);
router.delete('/users/:id', controller.user.destroy);
};
```
7. 启动应用
```bash
$ npm run dev
```
以上就是使用 Egg.js、TypeScript 和 MySQL 开发 Web 应用程序的基本步骤。
相关推荐
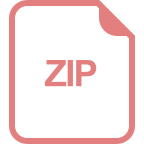
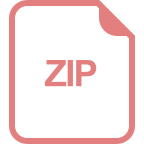
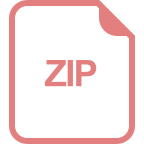
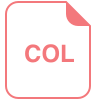













