pyqt开发的软件实现在线升级更新的代码例子
时间: 2023-10-26 10:04:02 浏览: 66
以下是一个使用PyQt实现在线升级更新的代码例子:
```python
from PyQt5.QtCore import QUrl, QFile, QIODevice, QCoreApplication
from PyQt5.QtGui import QIcon
from PyQt5.QtWidgets import QApplication, QMainWindow, QProgressBar, QPushButton, QMessageBox
from PyQt5.QtNetwork import QNetworkAccessManager, QNetworkRequest, QNetworkReply
class Updater(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle("Updater")
self.setWindowIcon(QIcon("icon.png"))
self.resize(400, 200)
self.progress_bar = QProgressBar(self)
self.progress_bar.setGeometry(30, 70, 340, 20)
self.update_button = QPushButton("Update", self)
self.update_button.setGeometry(150, 120, 100, 30)
self.update_button.clicked.connect(self.check_for_updates)
self.manager = QNetworkAccessManager(self)
self.download_file = None
def check_for_updates(self):
url = QUrl("http://example.com/latest_version.txt")
request = QNetworkRequest(url)
self.manager.get(request).finished.connect(self.handle_latest_version)
def handle_latest_version(self, reply):
latest_version = str(reply.readAll(), "utf-8").strip()
if latest_version != QCoreApplication.applicationVersion():
reply.deleteLater()
self.download_update()
else:
QMessageBox.information(self, "Updater", "You are already using the latest version.")
def download_update(self):
url = QUrl("http://example.com/latest_version.exe")
request = QNetworkRequest(url)
self.download_file = QFile("latest_version.exe")
if self.download_file.open(QIODevice.WriteOnly):
self.manager.get(request).finished.connect(self.handle_download)
else:
QMessageBox.warning(self, "Updater", "Could not write to update file.")
def handle_download(self, reply):
if reply.error() == QNetworkReply.NoError:
self.download_file.write(reply.readAll())
self.download_file.close()
self.install_update()
else:
QMessageBox.warning(self, "Updater", "Could not download update.")
def install_update(self):
QMessageBox.information(self, "Updater", "Update downloaded successfully. The application will now exit.")
QCoreApplication.exit()
if __name__ == "__main__":
app = QApplication([])
updater = Updater()
updater.show()
app.exec_()
```
这个例子的主要流程是:
1. 用户单击“Update”按钮,触发`check_for_updates`函数。
2. `check_for_updates`函数发送一个HTTP GET请求,获取最新版本的版本号。
3. `handle_latest_version`函数比较当前应用程序的版本号和最新版本的版本号,如果不同,则触发`download_update`函数。
4. `download_update`函数发送一个HTTP GET请求,下载最新版本的安装程序。
5. `handle_download`函数将下载的安装程序写入本地文件,如果下载错误,则显示警告。
6. `install_update`函数显示成功下载更新的消息,并退出应用程序。
注意,这个例子中的URL和文件名都是示例。在实际使用中,应该使用实际的URL和文件名。
相关推荐
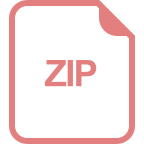
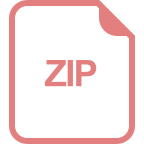














