pyqt开发的软件自动升级,安装包在B盘下,代码例子
时间: 2024-05-08 12:16:41 浏览: 115
以下是一个简单的代码示例,演示如何使用PyQt实现软件自动升级功能:
```
import sys
import os
import urllib.request
from PyQt5.QtCore import Qt, QThread, pyqtSignal
from PyQt5.QtWidgets import QApplication, QWidget, QLabel, QPushButton, QProgressBar, QMessageBox
class Updater(QWidget):
def __init__(self):
super().__init__()
self.title = "软件自动升级"
self.left = 100
self.top = 100
self.width = 400
self.height = 200
self.initUI()
def initUI(self):
self.setWindowTitle(self.title)
self.setGeometry(self.left, self.top, self.width, self.height)
self.label = QLabel(self)
self.label.setText("当前版本:1.0.0")
self.label.move(20, 20)
self.btn_check = QPushButton(self)
self.btn_check.setText("检查更新")
self.btn_check.move(20, 60)
self.btn_check.clicked.connect(self.check_update)
self.progress = QProgressBar(self)
self.progress.setGeometry(20, 100, 360, 20)
self.show()
def check_update(self):
# 检查更新版本号
url = "http://example.com/version.txt" # 更新版本号文件URL
try:
response = urllib.request.urlopen(url)
remote_version = response.read().decode().strip()
except:
QMessageBox.warning(self, "错误", "无法获取更新版本号")
return
# 比较当前版本和更新版本
current_version = "1.0.0" # 当前版本号
if remote_version == current_version:
QMessageBox.information(self, "提示", "当前已是最新版本")
else:
msg = "发现新版本:{}\n是否立即更新?".format(remote_version)
reply = QMessageBox.question(self, "更新", msg, QMessageBox.Yes | QMessageBox.No)
if reply == QMessageBox.Yes:
self.update()
def update(self):
self.btn_check.setEnabled(False)
self.progress.setValue(0)
self.thread = UpdateThread()
self.thread.start()
self.thread.update_progress.connect(self.update_progress)
def update_progress(self, percent):
self.progress.setValue(percent)
if percent == 100:
QMessageBox.information(self, "提示", "更新完成,请重启软件")
self.close()
class UpdateThread(QThread):
update_progress = pyqtSignal(int)
def run(self):
# 下载更新包
url = "http://example.com/update.zip" # 更新包URL
filename = "B:/update.zip" # 下载到本地路径
try:
urllib.request.urlretrieve(url, filename, reporthook=self.report_hook)
except:
QMessageBox.warning(None, "错误", "下载更新包失败")
return
# 解压更新包并替换旧文件
try:
import zipfile
with zipfile.ZipFile(filename, "r") as zip_ref:
zip_ref.extractall("B:/") # 解压到本地路径
except:
QMessageBox.warning(None, "错误", "解压更新包失败")
return
def report_hook(self, count, block_size, total_size):
percent = int(count * block_size * 100 / total_size)
self.update_progress.emit(percent)
if __name__ == "__main__":
app = QApplication(sys.argv)
updater = Updater()
sys.exit(app.exec_())
```
在这个例子中,我们创建了一个简单的GUI窗口,包括一个标签和一个按钮。点击按钮后,程序会检查更新版本号,如果有新版本则提示用户是否立即更新。如果用户确认更新,程序会启动一个后台线程,下载更新包并解压覆盖旧文件。在下载和解压的过程中,程序会更新进度条。更新完成后,程序会提示用户重启软件。
请注意,这个例子中的更新包和版本号文件的URL需要根据实际情况进行修改。另外,解压更新包会覆盖旧文件,所以请确保在更新包中只包含需要更新的文件。
阅读全文
相关推荐
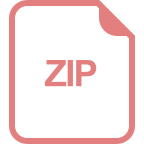
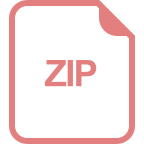
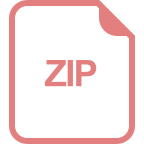















