Java实现NFA转DFA并给出使用示例
时间: 2024-02-04 12:02:24 浏览: 108
NFA(非确定有限状态自动机)是一种能够接受正则表达式描述的语言的自动机,而DFA(确定有限状态自动机)是一种处理有限长度字符串的自动机。将NFA转换为DFA可以使得语言的识别更高效,因为DFA比NFA更容易实现。
下面是一个Java实现NFA转DFA的示例代码:
```java
import java.util.*;
public class NFAtoDFA {
private Set<Integer> states;
private Set<Character> symbols;
private int initialState;
private Set<Integer> finalStates;
private Map<Integer, Map<Character, Set<Integer>>> transitionFunction;
public NFAtoDFA(Set<Integer> states, Set<Character> symbols, int initialState,
Set<Integer> finalStates, Map<Integer, Map<Character, Set<Integer>>> transitionFunction) {
this.states = states;
this.symbols = symbols;
this.initialState = initialState;
this.finalStates = finalStates;
this.transitionFunction = transitionFunction;
}
public DFA convertToDFA() {
Map<Set<Integer>, Integer> dfaStates = new HashMap<>();
List<Set<Integer>> dfaStateList = new ArrayList<>();
int stateCount = 0;
// Compute the epsilon closure of the initial state
Set<Integer> initialClosure = epsilonClosure(initialState);
// Add the initial state to the DFA
dfaStates.put(initialClosure, stateCount);
dfaStateList.add(initialClosure);
stateCount++;
// Initialize the transition function for the DFA
Map<Integer, Map<Character, Integer>> dfaTransitionFunction = new HashMap<>();
// Process each unmarked state in the list
for (int i = 0; i < dfaStateList.size(); i++) {
Set<Integer> dfaState = dfaStateList.get(i);
// For each symbol in the input alphabet
for (char symbol : symbols) {
// Compute the transition for this symbol
Set<Integer> transition = new HashSet<>();
for (int state : dfaState) {
Map<Character, Set<Integer>> transitionsForState = transitionFunction.get(state);
if (transitionsForState != null) {
Set<Integer> statesOnSymbol = transitionsForState.get(symbol);
if (statesOnSymbol != null) {
transition.addAll(statesOnSymbol);
}
}
}
// Compute the epsilon closure of the resulting state
Set<Integer> closure = epsilonClosure(transition);
// If the resulting state is new, add it to the DFA
if (!dfaStates.containsKey(closure)) {
dfaStates.put(closure, stateCount);
dfaStateList.add(closure);
stateCount++;
}
// Add the transition to the DFA transition function
int sourceState = dfaStates.get(dfaState);
int targetState = dfaStates.get(closure);
if (!dfaTransitionFunction.containsKey(sourceState)) {
dfaTransitionFunction.put(sourceState, new HashMap<>());
}
dfaTransitionFunction.get(sourceState).put(symbol, targetState);
}
}
// Compute the final states of the DFA
Set<Integer> dfaFinalStates = new HashSet<>();
for (Set<Integer> dfaState : dfaStateList) {
for (int nfaState : dfaState) {
if (finalStates.contains(nfaState)) {
dfaFinalStates.add(dfaStates.get(dfaState));
break;
}
}
}
// Create and return the DFA
return new DFA(dfaStateList.size(), symbols, 0, dfaFinalStates, dfaTransitionFunction);
}
private Set<Integer> epsilonClosure(int state) {
Set<Integer> closure = new HashSet<>();
Stack<Integer> stack = new Stack<>();
closure.add(state);
stack.push(state);
while (!stack.isEmpty()) {
int currentState = stack.pop();
Map<Character, Set<Integer>> transitionsForState = transitionFunction.get(currentState);
if (transitionsForState != null) {
Set<Integer> statesOnEpsilon = transitionsForState.get(null);
if (statesOnEpsilon != null) {
for (int nextState : statesOnEpsilon) {
if (!closure.contains(nextState)) {
closure.add(nextState);
stack.push(nextState);
}
}
}
}
}
return closure;
}
}
```
下面是一个使用示例:
```java
import java.util.*;
public class NFAtoDFAExample {
public static void main(String[] args) {
// Define the input alphabet
Set<Character> symbols = new HashSet<>();
symbols.add('a');
symbols.add('b');
// Define the NFA
Set<Integer> states = new HashSet<>(Arrays.asList(0, 1, 2, 3));
int initialState = 0;
Set<Integer> finalStates = new HashSet<>(Arrays.asList(3));
Map<Integer, Map<Character, Set<Integer>>> transitionFunction = new HashMap<>();
transitionFunction.put(0, new HashMap<>());
transitionFunction.get(0).put('a', new HashSet<>(Arrays.asList(0, 1)));
transitionFunction.get(0).put(null, new HashSet<>(Arrays.asList(2)));
transitionFunction.put(1, new HashMap<>());
transitionFunction.get(1).put('b', new HashSet<>(Arrays.asList(2)));
transitionFunction.put(2, new HashMap<>());
transitionFunction.get(2).put('a', new HashSet<>(Arrays.asList(3)));
// Convert the NFA to a DFA
NFAtoDFA nfaToDfa = new NFAtoDFA(states, symbols, initialState, finalStates, transitionFunction);
DFA dfa = nfaToDfa.convertToDFA();
// Test the DFA
System.out.println(dfa.accepts("aab")); // true
System.out.println(dfa.accepts("abb")); // false
}
}
```
在这个示例中,我们定义了一个NFA,并使用该NFA创建了一个NFAtoDFA对象。我们然后使用该对象调用convertToDFA()方法,该方法返回一个DFA对象。我们最后测试了DFA对象是否接受一些字符串。
阅读全文
相关推荐





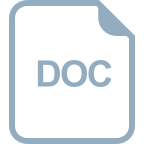

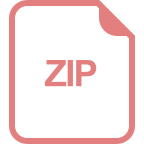
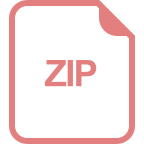









