用juputer notebook 选取表格里的内容
时间: 2024-04-29 09:23:06 浏览: 24
1. 首先需要导入 pandas 库,使用以下代码:
```
import pandas as pd
```
2. 读取表格数据,可以使用 pandas 的 read_csv 函数读取 csv 文件,或者 read_excel 函数读取 Excel 文件,例如:
```
data = pd.read_csv('data.csv')
```
3. 查看数据表格的内容,可以使用 head 函数查看前几行数据,或者使用 tail 函数查看后几行数据,例如:
```
data.head() # 查看前5行数据
data.tail() # 查看后5行数据
```
4. 选取表格里的内容,可以使用 loc 或者 iloc 函数,loc 函数按照行列的标签选取数据,iloc 函数按照行列的位置选取数据,例如:
```
# 选取第2列和第4列的数据
data.loc[:, ['列名2', '列名4']]
data.iloc[:, [1, 3]]
# 选取第3行和第5行的数据
data.loc[[2, 4], :]
data.iloc[[2, 4], :]
```
以上代码演示了如何在 Jupyter Notebook 中选取表格里的内容。
相关问题
jupyter notebook制作表格
Jupyter Notebook 可以使用 Markdown 语法制作表格。具体步骤如下:
1. 在 Jupyter Notebook 中新建一个 Markdown 单元格。
2. 在单元格中输入表格的内容,使用 Markdown 语法制作表格,例如:
```
| 姓名 | 年龄 | 性别 |
| --- | --- | --- |
| 张三 | 20 | 男 |
| 李四 | 22 | 女 |
```
3. 在单元格上方选择 Markdown 渲染器,将 Markdown 语法渲染为表格。
另外,Jupyter Notebook 还可以使用 Pandas 库制作表格,具体可以参考 Pandas 官方文档。
jupyter notebook创建表格
您可以使用Markdown语法在Jupyter Notebook中创建表格。以下是一个简单的示例:
```
| 姓名 | 年龄 | 性别 |
|------|------|------|
| 小明 | 20 | 男 |
| 小红 | 18 | 女 |
```
这将创建一个包含三列的表格,每列分别为“姓名”、“年龄”和“性别”,并在下面添加两行数据。
您可以根据需要添加或删除行和列,调整表格的大小和形状。请注意,表格中的每一行必须用管道符号“|”分隔,而每一列必须用连字符“-”分隔,以便正确显示表格。
另外,您可以使用HTML代码创建更复杂的表格,但这需要更高级的技能和知识。
相关推荐
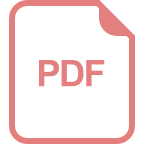
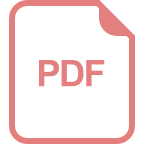
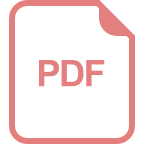












