用thinkphp5 写一个完整的客户管理系统(包含代码和数据)
时间: 2023-11-22 22:55:12 浏览: 30
很抱歉,我无法提供完整的代码和数据,但我可以提供一个大体的思路和代码示例。
首先,我们需要创建一个数据库,并创建一个名为 `customers` 的表,该表将存储客户的信息。表结构如下:
```sql
CREATE TABLE `customers` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`name` varchar(255) NOT NULL,
`email` varchar(255) NOT NULL,
`phone` varchar(20) NOT NULL,
`address` text NOT NULL,
`created_at` timestamp NOT NULL DEFAULT CURRENT_TIMESTAMP,
`updated_at` timestamp NOT NULL DEFAULT CURRENT_TIMESTAMP ON UPDATE CURRENT_TIMESTAMP,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
```
接下来,我们创建一个 `Customer` 模型,用于操作客户信息。在 `app` 目录下创建一个名为 `Customer.php` 的文件,代码如下:
```php
<?php
namespace app;
use think\Model;
class Customer extends Model
{
protected $table = 'customers';
}
```
然后,我们创建一个 `CustomerController` 控制器,用于处理客户相关的请求。在 `app` 目录下创建一个名为 `CustomerController.php` 的文件,代码如下:
```php
<?php
namespace app;
use think\Controller;
use think\Request;
class CustomerController extends Controller
{
// 客户列表页面
public function index()
{
$customers = Customer::all();
return view('index', ['customers' => $customers]);
}
// 添加客户页面
public function create()
{
return view('create');
}
// 保存客户信息
public function save(Request $request)
{
$customer = new Customer();
$customer->name = $request->post('name');
$customer->email = $request->post('email');
$customer->phone = $request->post('phone');
$customer->address = $request->post('address');
$customer->save();
return redirect('index');
}
// 编辑客户页面
public function edit($id)
{
$customer = Customer::get($id);
return view('edit', ['customer' => $customer]);
}
// 更新客户信息
public function update(Request $request, $id)
{
$customer = Customer::get($id);
$customer->name = $request->post('name');
$customer->email = $request->post('email');
$customer->phone = $request->post('phone');
$customer->address = $request->post('address');
$customer->save();
return redirect('index');
}
// 删除客户信息
public function delete($id)
{
$customer = Customer::get($id);
$customer->delete();
return redirect('index');
}
}
```
接下来,我们创建视图文件。在 `app/view` 目录下创建一个名为 `index.html` 的文件,代码如下:
```html
<!DOCTYPE html>
<html>
<head>
<title>客户列表</title>
</head>
<body>
<table>
<tr>
<th>ID</th>
<th>姓名</th>
<th>邮箱</th>
<th>电话</th>
<th>地址</th>
<th>操作</th>
</tr>
<?php foreach ($customers as $customer): ?>
<tr>
<td><?php echo $customer->id; ?></td>
<td><?php echo $customer->name; ?></td>
<td><?php echo $customer->email; ?></td>
<td><?php echo $customer->phone; ?></td>
<td><?php echo $customer->address; ?></td>
<td>
<a href="/customer/edit/<?php echo $customer->id; ?>">编辑</a>
<a href="/customer/delete/<?php echo $customer->id; ?>">删除</a>
</td>
</tr>
<?php endforeach; ?>
</table>
<a href="/customer/create">添加客户</a>
</body>
</html>
```
在 `app/view` 目录下创建一个名为 `create.html` 的文件,代码如下:
```html
<!DOCTYPE html>
<html>
<head>
<title>添加客户</title>
</head>
<body>
<form action="/customer/save" method="post">
<div>
<label>姓名:</label>
<input type="text" name="name">
</div>
<div>
<label>邮箱:</label>
<input type="text" name="email">
</div>
<div>
<label>电话:</label>
<input type="text" name="phone">
</div>
<div>
<label>地址:</label>
<textarea name="address"></textarea>
</div>
<div>
<button type="submit">保存</button>
</div>
</form>
</body>
</html>
```
在 `app/view` 目录下创建一个名为 `edit.html` 的文件,代码如下:
```html
<!DOCTYPE html>
<html>
<head>
<title>编辑客户</title>
</head>
<body>
<form action="/customer/update/<?php echo $customer->id; ?>" method="post">
<div>
<label>姓名:</label>
<input type="text" name="name" value="<?php echo $customer->name; ?>">
</div>
<div>
<label>邮箱:</label>
<input type="text" name="email" value="<?php echo $customer->email; ?>">
</div>
<div>
<label>电话:</label>
<input type="text" name="phone" value="<?php echo $customer->phone; ?>">
</div>
<div>
<label>地址:</label>
<textarea name="address"><?php echo $customer->address; ?></textarea>
</div>
<div>
<button type="submit">保存</button>
</div>
</form>
</body>
</html>
```
最后,我们需要定义路由。在 `app/route.php` 文件中添加以下代码:
```php
<?php
// 客户列表页面
Route::get('customer', 'CustomerController@index');
// 添加客户页面
Route::get('customer/create', 'CustomerController@create');
// 保存客户信息
Route::post('customer/save', 'CustomerController@save');
// 编辑客户页面
Route::get('customer/edit/:id', 'CustomerController@edit');
// 更新客户信息
Route::post('customer/update/:id', 'CustomerController@update');
// 删除客户信息
Route::get('customer/delete/:id', 'CustomerController@delete');
```
现在,我们就完成了一个简单的客户管理系统。
相关推荐
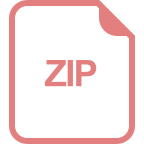
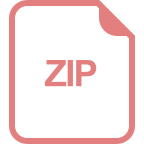
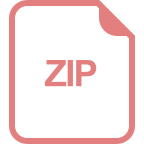
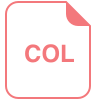
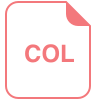
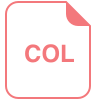
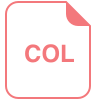
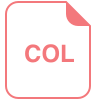









