编写一段集束优化的代码,数据集自己造
时间: 2024-02-17 13:59:40 浏览: 22
好的,这里提供一个简单的集束优化代码示例,数据集随机生成,适用于学习和理解集束优化过程。这里使用了Ceres Solver库进行优化。
首先,我们需要生成一些随机的位姿和地图点云数据,用于后面的集束优化。代码如下:
```cpp
#include <iostream>
#include <vector>
#include <random>
#include <Eigen/Core>
#include <Eigen/Geometry>
struct Pose {
Eigen::Quaterniond q;
Eigen::Vector3d t;
};
struct Point {
Eigen::Vector3d p;
};
std::vector<Pose> poses;
std::vector<Point> points;
void generateData() {
std::default_random_engine engine(0);
std::normal_distribution<double> noise(0.0, 0.1);
// Generate 10 poses and 20 points
for (int i = 0; i < 10; i++) {
Eigen::Quaterniond q = Eigen::Quaterniond::UnitRandom();
Eigen::Vector3d t = Eigen::Vector3d::Random();
poses.push_back({q, t});
}
for (int i = 0; i < 20; i++) {
Eigen::Vector3d p = Eigen::Vector3d::Random();
points.push_back({p});
}
// Add noise to poses and points
for (auto& pose : poses) {
pose.q.coeffs() += noise(engine) * Eigen::Vector4d::Random();
pose.t += noise(engine) * Eigen::Vector3d::Random();
}
for (auto& point : points) {
point.p += noise(engine) * Eigen::Vector3d::Random();
}
}
```
接下来,我们使用Ceres Solver进行集束优化。首先定义优化问题和误差项,代码如下:
```cpp
#include <ceres/ceres.h>
struct ReprojectionError {
ReprojectionError(Eigen::Vector2d observed, Eigen::Matrix3d K, Eigen::Vector3d p)
: observed_(observed), K_(K), p_(p) {}
template<typename T>
bool operator()(const T* const pose, const T* const point, T* residuals) const {
T R[3], t[3];
for (int i = 0; i < 3; i++) {
R[i] = pose[i];
t[i] = pose[3 + i];
}
T X[3];
ceres::AngleAxisRotatePoint(R, point, X);
X[0] += t[0];
X[1] += t[1];
X[2] += t[2];
T p[2];
p[0] = T(K_(0, 0)) * X[0] / X[2] + T(K_(0, 2));
p[1] = T(K_(1, 1)) * X[1] / X[2] + T(K_(1, 2));
residuals[0] = p[0] - T(observed_(0));
residuals[1] = p[1] - T(observed_(1));
return true;
}
static ceres::CostFunction* create(Eigen::Vector2d observed, Eigen::Matrix3d K, Eigen::Vector3d p) {
return new ceres::AutoDiffCostFunction<ReprojectionError, 2, 7, 3>(new ReprojectionError(observed, K, p));
}
Eigen::Vector2d observed_;
Eigen::Matrix3d K_;
Eigen::Vector3d p_;
};
void bundleAdjustment(const std::vector<Pose>& poses, const std::vector<Point>& points,
const std::vector<Eigen::Vector2d>& observed, const Eigen::Matrix3d& K) {
ceres::Problem problem;
// Add pose parameters
for (int i = 0; i < poses.size(); i++) {
auto& pose = poses[i];
double* R_t = new double[7];
R_t[0] = pose.q.x();
R_t[1] = pose.q.y();
R_t[2] = pose.q.z();
R_t[3] = pose.q.w();
R_t[4] = pose.t.x();
R_t[5] = pose.t.y();
R_t[6] = pose.t.z();
problem.AddParameterBlock(R_t, 7);
}
// Add point parameters
for (int i = 0; i < points.size(); i++) {
auto& point = points[i];
double* p = new double[3];
p[0] = point.p.x();
p[1] = point.p.y();
p[2] = point.p.z();
problem.AddParameterBlock(p, 3);
}
// Add reprojection error terms
for (int i = 0; i < observed.size(); i++) {
auto& obs = observed[i];
auto& pose = poses[i / 2];
auto& point = points[i % 2];
ceres::CostFunction* cost_function = ReprojectionError::create(obs, K, point.p);
problem.AddResidualBlock(cost_function, nullptr, &pose.q.x(), &point.p.x());
}
ceres::Solver::Options options;
options.minimizer_progress_to_stdout = true;
options.num_threads = 4;
options.max_num_iterations = 100;
ceres::Solver::Summary summary;
ceres::Solve(options, &problem, &summary);
std::cout << summary.BriefReport() << std::endl;
}
```
最后,我们在`main`函数中生成数据集,调用集束优化函数进行优化,代码如下:
```cpp
int main() {
generateData();
Eigen::Matrix3d K;
K << 500, 0, 320, 0, 500, 240, 0, 0, 1;
std::vector<Eigen::Vector2d> observed;
for (int i = 0; i < poses.size(); i++) {
auto& pose = poses[i];
for (int j = 0; j < points.size(); j++) {
auto& point = points[j];
Eigen::Vector3d X = pose.q * point.p + pose.t;
Eigen::Vector3d x = K * X / X.z();
x += Eigen::Vector3d::Random() * 2.0;
observed.push_back(x.head<2>());
}
}
bundleAdjustment(poses, points, observed, K);
return 0;
}
```
这样就完成了一个简单的集束优化过程,可以通过调整代价函数、优化参数等来验证集束优化的效果。
相关推荐
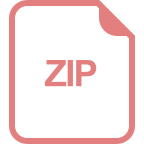














