使用C++解析3dl文件
时间: 2024-01-21 13:19:53 浏览: 32
解析3dl文件,需要先了解3dl文件的结构和格式。3dl文件是3D Studio Max软件使用的一种材质库文件,存储了材质、贴图、纹理等信息。
下面是解析3dl文件的大致思路:
1. 打开3dl文件,读取文件头信息和材质数量。
2. 逐个读取每个材质的信息,包括材质名称、贴图文件名、纹理信息等。
3. 对于每个材质,读取其贴图文件,并将其存储为纹理对象。
4. 将每个材质和对应的纹理对象存储到材质库中。
下面是一个简单的C++代码示例,用于读取3dl文件并输出材质名称、贴图文件名和纹理信息:
```cpp
#include <iostream>
#include <fstream>
#include <vector>
#include <string>
struct Texture {
std::string name;
int width;
int height;
unsigned char* data;
};
struct Material {
std::string name;
std::string texture_file;
Texture texture;
};
int main() {
std::ifstream file("material.3dl", std::ios::binary);
if (!file) {
std::cerr << "Failed to open file." << std::endl;
return -1;
}
// Read file header
int num_materials = 0;
file.read(reinterpret_cast<char*>(&num_materials), sizeof(num_materials));
// Read materials
std::vector<Material> materials(num_materials);
for (int i = 0; i < num_materials; ++i) {
Material& material = materials[i];
// Read material name
int name_length = 0;
file.read(reinterpret_cast<char*>(&name_length), sizeof(name_length));
material.name.resize(name_length);
file.read(&material.name[0], name_length);
// Read texture file name
int texture_file_length = 0;
file.read(reinterpret_cast<char*>(&texture_file_length), sizeof(texture_file_length));
material.texture_file.resize(texture_file_length);
file.read(&material.texture_file[0], texture_file_length);
// Read texture info
file.read(reinterpret_cast<char*>(&material.texture.width), sizeof(material.texture.width));
file.read(reinterpret_cast<char*>(&material.texture.height), sizeof(material.texture.height));
// Read texture data
int data_size = material.texture.width * material.texture.height * 3;
material.texture.data = new unsigned char[data_size];
file.read(reinterpret_cast<char*>(material.texture.data), data_size);
// Print material info
std::cout << "Material name: " << material.name << std::endl;
std::cout << "Texture file: " << material.texture_file << std::endl;
std::cout << "Texture width: " << material.texture.width << std::endl;
std::cout << "Texture height: " << material.texture.height << std::endl;
}
// Clean up
for (int i = 0; i < num_materials; ++i) {
delete[] materials[i].texture.data;
}
return 0;
}
```
注意:上述示例仅用于演示读取3dl文件的基本过程,实际应用中需要进行错误检查和异常处理等操作。
相关推荐
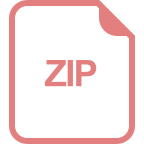
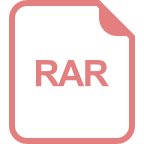









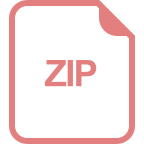
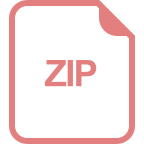
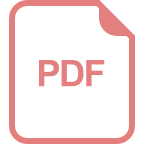
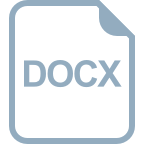
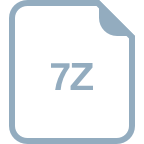