string转double c++
时间: 2023-05-04 22:06:09 浏览: 153
在C语言中,将字符串转换为双精度浮点数需要使用库函数atof()。在使用之前,需要在程序中添加头文件<stdlib.h>。
atof()函数的原型如下:
double atof(const char *str);
该函数接受一个指向字符串的指针,并返回相应的双精度浮点数。其中,字符串必须以数字开头,并可以包含以科学计数法表示的十六进制数字、负号和小数点。
使用atof()函数进行字符串转换的示例代码如下:
#include <stdlib.h>
#include <stdio.h>
int main()
{
char str[] = "3.14159";
double dval = atof(str);
printf("%lf\n", dval);
return 0;
}
在上述代码中,字符串"3.14159"被转换为双精度浮点数dval,并使用printf()函数输出其值。
需要注意的是,如果字符串无法转换为双精度浮点数,atof()函数将返回0.0。因此,在程序中应该先使用函数如isdigit()等判断字符串是否符合转换条件,避免因转换错误导致的程序异常。
总之,在C语言中将字符串转换为双精度浮点数需要使用atof()函数,并注意检测转换前字符串的合法性。
相关问题
string转double C++
将std::string转换为double的方法有几种。一种方法是使用自定义函数convertFromString,它接受一个double变量和一个std::string作为参数,并将std::string转换为double。另一种方法是使用stringstream,将std::string转换为一个临时的stringstream对象,然后使用该对象的>>运算符将其转换为double。
以下是使用convertFromString函数将std::string转换为double的示例代码:
```cpp
#include <iostream>
#include <sstream>
template<typename T>
void convertFromString(T& value, const std::string& str) {
std::stringstream ss(str);
ss >> value;
}
int main() {
std::string str = "3.14";
double d = 0;
convertFromString(d, str);
std::cout << d << std::endl;
return 0;
}
```
使用stringstream将std::string转换为double的示例代码如下:
```cpp
#include <iostream>
#include <sstream>
int main() {
std::string str = "3.14";
std::stringstream ss(str);
double d = 0;
ss >> d;
std::cout << d << std::endl;
return 0;
}
```
c++ string 转 double
可以使用 atof() 函数将 C 字符串转换为 double 类型。
例如:
```c
#include <stdio.h>
#include <stdlib.h>
int main() {
char str[] = "3.14159";
double num = atof(str);
printf("num = %f\n", num);
return 0;
}
```
此程序将输出:
```
num = 3.141590
```
阅读全文
相关推荐
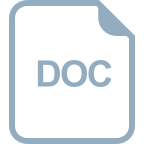
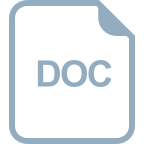
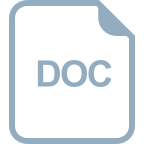
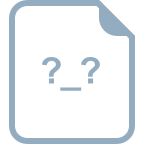












