vue怎么实现游戏摇杆
时间: 2024-01-30 18:03:59 浏览: 72
要在 Vue 中实现游戏摇杆,可以考虑以下步骤:
1. 创建一个组件,用于显示游戏摇杆和响应用户操作。
2. 在组件中,可以使用 `canvas` 元素绘制游戏摇杆的外框和摇杆。
3. 在 `mounted` 钩子函数中,监听 `canvas` 元素的 `touchstart`、`touchmove`、`touchend` 事件,根据用户手指的操作,计算出摇杆的位置和偏移量。
4. 在 `touchmove` 事件中,需要不断重新绘制摇杆的位置,以及根据位置计算出摇杆的偏移量。
5. 在组件内定义一个 `joystick` 对象,用于保存当前摇杆的位置和偏移量,可以通过 `props` 属性将其传递给父组件。
6. 在 `touchend` 事件中,需要重置 `joystick` 对象的位置和偏移量。
下面是一个简单的 Vue 游戏摇杆组件示例代码:
```html
<template>
<div>
<canvas ref="canvas" />
</div>
</template>
<script>
export default {
props: {
radius: {
type: Number,
default: 50
},
bgColor: {
type: String,
default: '#eee'
},
fgColor: {
type: String,
default: '#999'
}
},
data() {
return {
joystick: {
x: 0,
y: 0,
dx: 0,
dy: 0
}
}
},
mounted() {
this.canvas = this.$refs.canvas
this.ctx = this.canvas.getContext('2d')
this.canvas.width = this.radius * 2
this.canvas.height = this.radius * 2
this.canvas.addEventListener('touchstart', this.touchStart)
this.canvas.addEventListener('touchmove', this.touchMove)
this.canvas.addEventListener('touchend', this.touchEnd)
this.draw()
},
methods: {
draw() {
this.ctx.clearRect(0, 0, this.canvas.width, this.canvas.height)
// 绘制背景圆
this.ctx.beginPath()
this.ctx.arc(this.radius, this.radius, this.radius, 0, 2 * Math.PI)
this.ctx.fillStyle = this.bgColor
this.ctx.fill()
// 绘制摇杆圆
this.ctx.beginPath()
this.ctx.arc(
this.radius + this.joystick.dx,
this.radius + this.joystick.dy,
this.radius / 2,
0,
2 * Math.PI
)
this.ctx.fillStyle = this.fgColor
this.ctx.fill()
requestAnimationFrame(this.draw)
},
touchStart(e) {
e.preventDefault()
const rect = this.canvas.getBoundingClientRect()
const touch = e.touches[0]
this.joystick.x = touch.clientX - rect.left
this.joystick.y = touch.clientY - rect.top
},
touchMove(e) {
e.preventDefault()
const rect = this.canvas.getBoundingClientRect()
const touch = e.touches[0]
const x = touch.clientX - rect.left
const y = touch.clientY - rect.top
const dx = x - this.joystick.x
const dy = y - this.joystick.y
const maxDist = this.radius / 2
if (Math.sqrt(dx * dx + dy * dy) > maxDist) {
const angle = Math.atan2(dy, dx)
this.joystick.dx = maxDist * Math.cos(angle)
this.joystick.dy = maxDist * Math.sin(angle)
} else {
this.joystick.dx = dx
this.joystick.dy = dy
}
},
touchEnd(e) {
e.preventDefault()
this.joystick.dx = 0
this.joystick.dy = 0
}
}
}
</script>
```
在父组件中,可以使用 `<game-joystick>` 标签引入游戏摇杆组件,并通过 `props` 属性设置游戏摇杆的半径、背景色和前景色。
```html
<template>
<div>
<game-joystick
:radius="50"
bgColor="#eee"
fgColor="#999"
@joystickmove="onJoystickMove"
/>
</div>
</template>
<script>
import GameJoystick from './GameJoystick.vue'
export default {
components: {
GameJoystick
},
methods: {
onJoystickMove(joystick) {
// 处理游戏摇杆移动事件
}
}
}
</script>
```
相关推荐
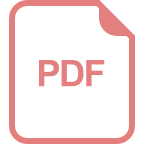
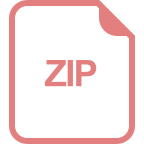














