给我一个bisenetv2 pytorcfh代码用来图像分割的案例
时间: 2023-06-15 10:03:15 浏览: 115
以下是一个基于 PyTorch 的 BiSeNetv2 图像分割案例代码:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
class ConvBlock(nn.Module):
def __init__(self, in_channels, out_channels, kernel_size=3, stride=1, padding=1, dilation=1, groups=1, bias=False):
super(ConvBlock, self).__init__()
self.conv = nn.Conv2d(in_channels, out_channels, kernel_size, stride=stride, padding=padding, dilation=dilation, groups=groups, bias=bias)
self.bn = nn.BatchNorm2d(out_channels)
self.relu = nn.ReLU(inplace=True)
def forward(self, x):
x = self.conv(x)
x = self.bn(x)
x = self.relu(x)
return x
class SpatialPath(nn.Module):
def __init__(self):
super(SpatialPath, self).__init__()
self.conv1 = ConvBlock(3, 64, kernel_size=3, stride=2, padding=1)
self.conv2 = ConvBlock(64, 128, kernel_size=3, stride=2, padding=1)
self.conv3 = ConvBlock(128, 256, kernel_size=3, stride=2, padding=1)
def forward(self, x):
x = self.conv1(x)
x = self.conv2(x)
x = self.conv3(x)
return x
class AttentionRefinement(nn.Module):
def __init__(self, in_channels, out_channels):
super(AttentionRefinement, self).__init__()
self.conv = ConvBlock(in_channels, out_channels, kernel_size=1, stride=1, padding=0)
self.sigmoid = nn.Sigmoid()
def forward(self, x):
y = F.adaptive_avg_pool2d(x, output_size=(1,1))
y = self.conv(y)
y = self.sigmoid(y)
return x * y
class ContextPath(nn.Module):
def __init__(self):
super(ContextPath, self).__init__()
self.conv1 = ConvBlock(3, 64, kernel_size=3, stride=2, padding=1)
self.conv2 = ConvBlock(64, 64, kernel_size=3, stride=1, padding=1)
self.conv3 = ConvBlock(64, 128, kernel_size=3, stride=2, padding=1)
self.conv4 = ConvBlock(128, 128, kernel_size=3, stride=1, padding=1, dilation=2)
self.conv5 = ConvBlock(128, 128, kernel_size=3, stride=1, padding=1, dilation=4)
self.conv6 = ConvBlock(128, 128, kernel_size=3, stride=1, padding=1, dilation=8)
self.conv7 = ConvBlock(128, 128, kernel_size=3, stride=1, padding=1, dilation=16)
self.conv8 = ConvBlock(128, 256, kernel_size=1, stride=1, padding=0)
self.arm = AttentionRefinementModule(256, 256)
def forward(self, x):
x = self.conv1(x)
x = self.conv2(x)
x = self.conv3(x)
x = self.conv4(x)
x = self.conv5(x)
x = self.conv6(x)
x = self.conv7(x)
x = self.conv8(x)
x = self.arm(x)
return x
class AttentionRefinementModule(nn.Module):
def __init__(self, in_channels, out_channels):
super(AttentionRefinementModule, self).__init__()
self.conv = ConvBlock(in_channels, out_channels, kernel_size=1, stride=1, padding=0)
self.arm = AttentionRefinement(in_channels, out_channels)
def forward(self, x):
x = self.conv(x)
x = self.arm(x)
return x
class FeatureFusionModule(nn.Module):
def __init__(self, in_channels, out_channels, reduction=1):
super(FeatureFusionModule, self).__init__()
self.conv1 = ConvBlock(in_channels, out_channels, kernel_size=1, stride=1, padding=0)
self.conv2 = ConvBlock(out_channels, out_channels, kernel_size=3, stride=1, padding=1)
self.conv3 = ConvBlock(out_channels, out_channels, kernel_size=1, stride=1, padding=0)
self.arm = AttentionRefinement(out_channels, out_channels//reduction)
def forward(self, x1, x2):
x = torch.cat([x1, x2], dim=1)
x = self.conv1(x)
x = self.conv2(x)
x = self.conv3(x)
x = self.arm(x)
x = x1 + x
return x
class BiSeNetv2(nn.Module):
def __init__(self, num_classes):
super(BiSeNetv2, self).__init__()
self.sp = SpatialPath()
self.cp = ContextPath()
self.ffm = FeatureFusionModule(256, 256, reduction=4)
self.conv = ConvBlock(256, num_classes, kernel_size=1, stride=1, padding=0)
self.softmax = nn.Softmax(dim=1)
def forward(self, x):
sp = self.sp(x)
cp = self.cp(x)
x = self.ffm(sp, cp)
x = self.conv(x)
x = self.softmax(x)
return x
```
使用该模型进行图像分割需要进行以下步骤:
1. 定义模型
```python
model = BiSeNetv2(num_classes)
```
2. 定义损失函数
```python
criterion = nn.CrossEntropyLoss()
```
3. 定义优化器
```python
optimizer = torch.optim.Adam(model.parameters(), lr=lr)
```
4. 训练模型
```python
for epoch in range(num_epochs):
for inputs, labels in dataloader:
inputs, labels = inputs.cuda(), labels.cuda()
optimizer.zero_grad()
outputs = model(inputs)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
```
其中,`dataloader` 是用于加载训练数据的 PyTorch 数据加载器,`num_epochs` 是训练轮数,`lr` 是学习率。
5. 对测试集进行预测
```python
model.eval()
with torch.no_grad():
for inputs, labels in test_dataloader:
inputs, labels = inputs.cuda(), labels.cuda()
outputs = model(inputs)
preds = torch.argmax(outputs, dim=1)
```
其中,`test_dataloader` 是用于加载测试数据的 PyTorch 数据加载器。`preds` 是预测的分割结果。
阅读全文
相关推荐
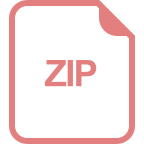
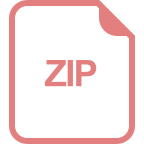
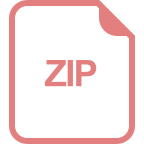
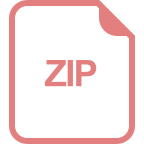
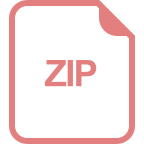
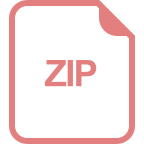
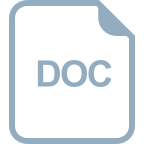








