用c语言实现带括号的四则运算
时间: 2023-10-04 18:08:31 浏览: 140
以下是一个简单的 C 语言程序,可以实现带括号的四则运算:
```c
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
#define MAXSIZE 100
typedef struct {
int top;
char data[MAXSIZE];
} Stack;
void push(Stack *s, char c) {
if (s->top == MAXSIZE - 1) {
printf("Stack overflow\n");
exit(1);
}
s->data[++s->top] = c;
}
char pop(Stack *s) {
if (s->top == -1) {
printf("Stack underflow\n");
exit(1);
}
return s->data[s->top--];
}
int priority(char c) {
switch (c) {
case '+':
case '-':
return 1;
case '*':
case '/':
return 2;
case '(':
return 0;
default:
printf("Invalid operator: %c\n", c);
exit(1);
}
}
int is_operator(char c) {
return (c == '+' || c == '-' || c == '*' || c == '/');
}
int is_operand(char c) {
return isdigit(c);
}
void infix_to_postfix(char *infix, char *postfix) {
Stack s;
s.top = -1;
int i = 0, j = 0;
while (infix[i] != '\0') {
if (is_operand(infix[i])) {
postfix[j++] = infix[i++];
} else if (is_operator(infix[i])) {
while (s.top != -1 && priority(s.data[s.top]) >= priority(infix[i])) {
postfix[j++] = pop(&s);
}
push(&s, infix[i++]);
} else if (infix[i] == '(') {
push(&s, infix[i++]);
} else if (infix[i] == ')') {
while (s.data[s.top] != '(') {
postfix[j++] = pop(&s);
}
pop(&s);
i++;
} else {
printf("Invalid character: %c\n", infix[i]);
exit(1);
}
}
while (s.top != -1) {
postfix[j++] = pop(&s);
}
postfix[j] = '\0';
}
int evaluate_postfix(char *postfix) {
Stack s;
s.top = -1;
int i = 0;
while (postfix[i] != '\0') {
if (is_operand(postfix[i])) {
push(&s, postfix[i] - '0');
} else if (is_operator(postfix[i])) {
int op2 = pop(&s);
int op1 = pop(&s);
switch (postfix[i]) {
case '+':
push(&s, op1 + op2);
break;
case '-':
push(&s, op1 - op2);
break;
case '*':
push(&s, op1 * op2);
break;
case '/':
push(&s, op1 / op2);
break;
default:
printf("Invalid operator: %c\n", postfix[i]);
exit(1);
}
} else {
printf("Invalid character: %c\n", postfix[i]);
exit(1);
}
i++;
}
return pop(&s);
}
int main() {
char infix[MAXSIZE], postfix[MAXSIZE];
printf("Enter an infix expression: ");
scanf("%s", infix);
infix_to_postfix(infix, postfix);
printf("Postfix expression: %s\n", postfix);
printf("Result: %d\n", evaluate_postfix(postfix));
return 0;
}
```
上面的程序使用了栈来实现中缀表达式转后缀表达式和后缀表达式求值的功能。在转换中缀表达式为后缀表达式的过程中,需要注意运算符的优先级和括号的匹配。
阅读全文
相关推荐
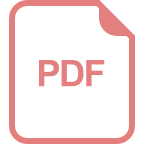
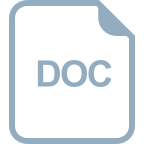






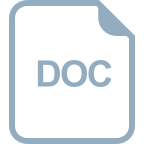
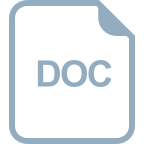
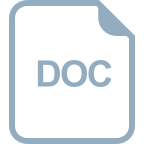





