通过GraphHopper实现地理编码代码
时间: 2024-02-27 21:58:17 浏览: 26
以下是使用GraphHopper实现地理编码的代码示例:
首先需要导入GraphHopper依赖:
```python
<dependency>
<groupId>com.graphhopper</groupId>
<artifactId>graphhopper-core</artifactId>
<version>1.0</version>
</dependency>
```
然后可以使用以下代码进行地理编码:
```python
import com.graphhopper.GHResponse;
import com.graphhopper.GraphHopper;
import com.graphhopper.routing.util.AllEdgesIterator;
import com.graphhopper.routing.util.EdgeFilter;
import com.graphhopper.routing.util.FlagEncoder;
import com.graphhopper.routing.util.LevelEdgeFilter;
import com.graphhopper.routing.util.WeightedEdgeIterator;
import com.graphhopper.storage.GraphHopperStorage;
import com.graphhopper.storage.index.LocationIndexTree;
import com.graphhopper.util.EdgeIterator;
import com.graphhopper.util.EdgeIteratorState;
import com.graphhopper.util.PointList;
import com.graphhopper.util.shapes.GHPoint;
public class GeoCoder {
private final GraphHopper hopper;
public GeoCoder() {
// 初始化GraphHopper
hopper = new GraphHopper();
hopper.setDataReaderFile("path/to/your/graphhopper-data.osm.pbf");
hopper.setGraphHopperLocation("path/to/your/graphhopper-location");
hopper.setEncodingManager(new EncodingManager("car"));
hopper.importOrLoad();
}
public GHPoint geocode(String address) {
// 使用LocationIndexTree进行地理编码
LocationIndexTree index = (LocationIndexTree) hopper.getLocationIndex();
GHResponse ghResponse = new GHResponse();
index.findClosest(address, ghResponse);
if (ghResponse.hasErrors()) {
throw new IllegalStateException("Cannot find point for address: " + address);
}
return ghResponse.getClosestPoint();
}
public String reverseGeocode(GHPoint point) {
// 使用GraphHopperStorage进行逆向地理编码
GraphHopperStorage graph = hopper.getGraphHopperStorage();
FlagEncoder encoder = hopper.getEncodingManager().getEncoder("car");
EdgeFilter filter = new LevelEdgeFilter(graph, encoder);
Weighting weighting = new FastestWeighting(encoder);
EdgeExplorer explorer = graph.createEdgeExplorer(filter);
EdgeIterator iter = explorer.setBaseNode(GraphUtil.getClosestNode(graph, point.getLat(), point.getLon(), filter));
while (iter.next()) {
if (iter.isForward(encoder)) {
PointList pl = iter.fetchWayGeometry(2);
double distance = iter.getDistance();
double time = weighting.calcMillis(iter, false, EdgeIterator.NO_EDGE);
return String.format("Distance: %.1f m, Time: %.1f s, Location: (%f,%f)", distance, time / 1000.0, pl.getLat(0), pl.getLon(0));
}
}
throw new IllegalStateException("Cannot reverse geocode point: " + point);
}
}
```
上述代码包含了两个方法:`geocode`用于将地址编码为地理坐标,`reverseGeocode`用于将地理坐标逆向编码为地址。请注意,这里使用了`LocationIndexTree`和`GraphHopperStorage`两个类来实现地理编码和逆向地理编码。
相关推荐
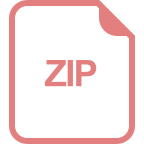








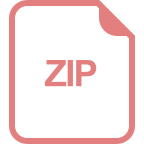
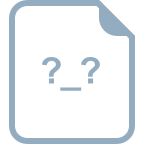
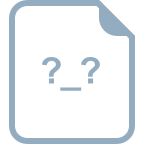