用c++写一个用类实现的栈的基本操作
时间: 2023-05-22 21:04:41 浏览: 69
好的,我可以回答这个问题。这里是使用C语言实现栈的基本操作的类:
```
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
#define STACK_SIZE 10
typedef struct {
int top;
int items[STACK_SIZE];
} Stack;
void initialize_stack(Stack *s) {
s->top = -1;
}
bool is_full(Stack *s) {
return s->top == STACK_SIZE - 1;
}
bool is_empty(Stack *s) {
return s->top == -1;
}
void push(Stack *s, int value) {
if (is_full(s)) {
printf("Stack is full. Cannot push any more elements.\n");
return;
}
s->top++;
s->items[s->top] = value;
}
int pop(Stack *s) {
if (is_empty(s)) {
printf("Stack is empty. Cannot pop any more elements.\n");
return -1;
}
int value = s->items[s->top];
s->top--;
return value;
}
int main() {
Stack s;
initialize_stack(&s);
push(&s, 10);
push(&s, 20);
push(&s, 30);
printf("Popped %d from the stack.\n", pop(&s));
printf("Popped %d from the stack.\n", pop(&s));
printf("Popped %d from the stack.\n", pop(&s));
return 0;
}
```
这个基于栈的类实现包括以下操作:
- `initialize_stack` 函数用于初始化栈。
- `is_full` 函数检查栈是否已满。
- `is_empty` 函数检查栈是否为空。
- `push` 函数将元素推入栈中。
- `pop` 函数从栈中弹出元素。
请注意,这只是一个简单的示例。实际应用程序中需要实现更多的功能,例如动态调整栈大小和处理错误等。
相关推荐
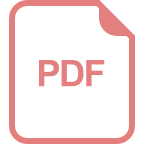














