计算平均成绩并统计低于平均分的学生成绩与人数
时间: 2024-12-17 18:41:39 浏览: 6
为了计算平均成绩并统计低于平均分的学生成绩与人数,你需要遍历学生分数数组,计算总分,然后除以学生数量得到平均分。接下来,你可以再次遍历数组,检查每个分数是否低于平均分,如果是,就增加计数器并将该分数加入到低于平均分的成绩列表中。这里是一个简单的C++代码示例:
```cpp
#include <iostream>
#include <vector>
// 假设我们有一个名为`StudentScore`的结构体或类,包含学生的姓名和分数
struct StudentScore {
std::string name;
float score;
};
int main() {
// 假设这是学生的分数数据
std::vector<StudentScore> scores = {
{"Alice", 85},
{"Bob", 90},
{"Charlie", 75},
{"Dave", 88},
{"Eve", 92}
};
int total_students = scores.size();
float sum_scores = 0;
// 计算总分
for (const auto& score : scores) {
sum_scores += score.score;
}
// 计算平均分
float average_score = sum_scores / total_students;
// 初始化计数器和低于平均分的成绩列表
int below_average_count = 0;
std::vector<float> below_average_scores;
// 遍历数组,统计低于平均分的学生成绩和数量
for (const auto& score : scores) {
if (score.score < average_score) {
below_average_count++;
below_average_scores.push_back(score.score);
}
}
// 输出结果
std::cout << "Average Score: " << average_score << std::endl;
std::cout << "Number of students with scores below the average: " << below_average_count << std::endl;
std::cout << "Scores below the average: ";
for (float s : below_average_scores) {
std::cout << s << " ";
}
std::cout << std::endl;
return 0;
}
```
阅读全文
相关推荐
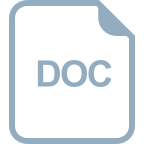
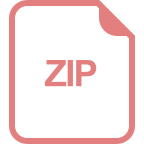
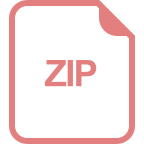















