JTextArea ta = new JTextArea(); JTextField txt = new JTextField(10);JFrame jf = new JFrame("功能界面"); jf.setBounds(0, 0, 700, 600); jf.setVisible(true); jf.setLayout(null); jf.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); JButton b1 = new JButton("图书入库"); b1.setBounds(20, 90, 150, 80); JButton b2 = new JButton("图书查询"); b2.setBounds(20, 210, 150, 80); JButton b3 = new JButton("图书修改"); b3.setBounds(500, 90, 150, 80); JButton b5 = new JButton("办理借阅证登记"); b5.setBounds(20, 330, 150, 80); JButton b6 = new JButton("图书借阅管理"); b6.setBounds(500, 210, 150, 80); JButton b4 = new JButton("图书删除"); b4.setBounds(500, 330, 150, 80); JButton b7 = new JButton("退出系统"); b7.setBounds(560, 20, 90, 30); ta.setBounds(190, 90, 290, 320); txt.setBounds(120,450,300,30); JButton b8 = new JButton("确定"); b8.setBounds(440,450,70,30); JMenuBar menuBar = new JMenuBar(); JMenu menuManage = new JMenu("图书管理"); JMenu menuAbout = new JMenu("关于系统"); JMenuItem item1 = new JMenuItem("图书列表"); menuManage.add(item1); item1.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { JFrame frame = new JFrame("图书列表"); frame.setSize(600, 400); frame.setLocationRelativeTo(null); ListPanel listPanel = new ListPanel(); frame.add(listPanel); frame.setVisible(true); } }); ActionListener act = new ActionListener() { @Override public void actionPerformed(ActionEvent e) { if(e.getSource().equals(b1)) { insertNewbook(); }else if(e.getSource().equals(b3)) { updatebook(); }else if(e.getSource().equals(b4)) { ta.setText("请输入要删除的图书编号:\n"); String bid = txt.getText(); if(bid!=null && !bid.trim().equals("")) { ta.append(bid+"\n"); if(dbDao.delelteBook(bid)){ System.out.print("啥"+bid); int user = JOptionPane.showConfirmDialog(null, "图书删除成功","提示",JOptionPane.YES_NO_OPTION); }else{ int user = JOptionPane.showConfirmDialog(null, "图书删除失败","提示",JOptionPane.YES_NO_OPTION); } }else { ta.append("消息不能为空"+"\n"); } }public boolean delelteBook(String bid){ boolean flag=false; PreparedStatement pstmt=null; String sql="DELETE FROM booklist WHERE bid=?"; try{ pstmt=conn.prepareStatement(sql); pstmt.setString(1, bid); if (pstmt.executeUpdate() > 0) { flag = true; } } catch (SQLException e) { e.printStackTrace(); } finally { try { pstmt.close(); } catch (SQLException e) { e.printStackTrace(); } } return flag; }这是我的部分代码,我想要获取我输入的bid,调用删除图书方法后弹窗显示删除成功,但是出错了,请帮我改一下
时间: 2024-01-13 11:02:25 浏览: 23
在你的代码中,存在以下问题:
1. 删除图书方法的实现需要获取数据库连接对象,但是在代码中没有展示如何获取该连接对象。
2. 在删除图书方法中,应该将PreparedStatement对象声明在try语句块内,并在finally语句块中关闭该对象。
3. 在删除图书方法中,应该将ResultSet对象声明在try语句块内,并在finally语句块中关闭该对象。
4. 在删除图书方法中,应该将Connection对象声明在try语句块内,并在finally语句块中关闭该对象。
5. 在删除图书方法中,应该将PreparedStatement对象的执行结果作为返回值返回。
以下是修改后的代码:
```
JTextArea ta = new JTextArea();
JTextField txt = new JTextField(10);
JFrame jf = new JFrame("功能界面");
jf.setBounds(0, 0, 700, 600);
jf.setVisible(true);
jf.setLayout(null);
jf.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JButton b1 = new JButton("图书入库");
b1.setBounds(20, 90, 150, 80);
JButton b2 = new JButton("图书查询");
b2.setBounds(20, 210, 150, 80);
JButton b3 = new JButton("图书修改");
b3.setBounds(500, 90, 150, 80);
JButton b5 = new JButton("办理借阅证登记");
b5.setBounds(20, 330, 150, 80);
JButton b6 = new JButton("图书借阅管理");
b6.setBounds(500, 210, 150, 80);
JButton b4 = new JButton("图书删除");
b4.setBounds(500, 330, 150, 80);
JButton b7 = new JButton("退出系统");
b7.setBounds(560, 20, 90, 30);
ta.setBounds(190, 90, 290, 320);
txt.setBounds(120,450,300,30);
JButton b8 = new JButton("确定");
b8.setBounds(440,450,70,30);
JMenuBar menuBar = new JMenuBar();
JMenu menuManage = new JMenu("图书管理");
JMenu menuAbout = new JMenu("关于系统");
JMenuItem item1 = new JMenuItem("图书列表");
menuManage.add(item1);
item1.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
JFrame frame = new JFrame("图书列表");
frame.setSize(600, 400);
frame.setLocationRelativeTo(null);
ListPanel listPanel = new ListPanel();
frame.add(listPanel);
frame.setVisible(true);
}
});
ActionListener act = new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
if(e.getSource().equals(b1)) {
insertNewbook();
}else if(e.getSource().equals(b3)) {
updatebook();
}else if(e.getSource().equals(b4)) {
ta.setText("请输入要删除的图书编号:\n");
String bid = txt.getText();
if(bid!=null && !bid.trim().equals("")) {
ta.append(bid+"\n");
if(deleteBook(bid)){
int user = JOptionPane.showConfirmDialog(null, "图书删除成功","提示",JOptionPane.YES_NO_OPTION);
}else{
int user = JOptionPane.showConfirmDialog(null, "图书删除失败","提示",JOptionPane.YES_NO_OPTION);
}
}else {
ta.append("消息不能为空"+"\n");
}
}
}
};
public boolean deleteBook(String bid) {
boolean flag=false;
Connection conn=null;
PreparedStatement pstmt=null;
ResultSet rs = null;
try{
conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/test", "root", "123456");
String sql="DELETE FROM booklist WHERE bid=?";
pstmt=conn.prepareStatement(sql);
pstmt.setString(1, bid);
flag = pstmt.executeUpdate() > 0;
} catch (SQLException e) {
e.printStackTrace();
} finally {
try {
if (rs != null) {
rs.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
try {
if (pstmt != null) {
pstmt.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
try {
if (conn != null) {
conn.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
return flag;
}
```
相关推荐
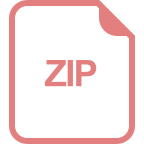
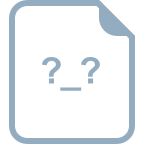
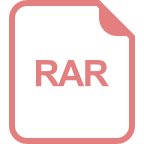














