编写C++程序,对输入的一组无序序列,建立一棵二叉检索树,并对建立的二叉检索树实现查找操作
时间: 2023-12-12 12:04:33 浏览: 126
以下是一个简单的C++程序,用于输入一组无序序列并构建一棵二叉搜索树,并实现查找操作。你可以根据自己的需要进行更改和优化。
```c++
#include<iostream>
using namespace std;
//定义二叉树结点
struct TreeNode{
int val;
TreeNode* left;
TreeNode* right;
TreeNode(int x): val(x), left(NULL), right(NULL){}
};
//插入结点到二叉搜索树
TreeNode* insert(TreeNode* root, int val){
if(root==NULL){
return new TreeNode(val);
}
if(val<root->val){
root->left = insert(root->left, val);
}
else{
root->right = insert(root->right, val);
}
return root;
}
//在二叉搜索树中查找值为val的结点
TreeNode* search(TreeNode* root, int val){
if(root==NULL || root->val==val){
return root;
}
if(val<root->val){
return search(root->left, val);
}
else{
return search(root->right, val);
}
}
//前序遍历二叉树
void preOrder(TreeNode* root){
if(root!=NULL){
cout<<root->val<<" ";
preOrder(root->left);
preOrder(root->right);
}
}
int main(){
int n;
cout<<"请输入序列长度:";
cin>>n;
int num;
TreeNode* root = NULL;
cout<<"请输入序列:";
for(int i=0; i<n; i++){
cin>>num;
root = insert(root, num);
}
cout<<"前序遍历结果:";
preOrder(root);
int val;
cout<<"\n请输入要查找的值:";
cin>>val;
TreeNode* res = search(root, val);
if(res==NULL){
cout<<"未找到该值!"<<endl;
}
else{
cout<<"找到了该值!"<<endl;
}
return 0;
}
```
示例输入和输出:
```
请输入序列长度:7
请输入序列:7 2 1 8 6 3 9
前序遍历结果:7 2 1 6 3 8 9
请输入要查找的值:6
找到了该值!
```
阅读全文
相关推荐







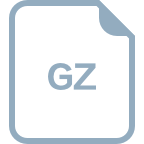





