如何在Codesys中解析json 文件
时间: 2024-05-28 19:12:35 浏览: 353
Codesys提供了Json库,可以用于解析和处理Json文件。以下是一个简单的步骤:
1. 在Codesys中创建一个新的程序。
2. 在程序中添加Json库。可以通过“库管理器”中的“添加库”选项来添加。
3. 创建一个新的函数块,并将Json库中的“JsonParser”函数块添加到该函数块中。
4. 在函数块中定义一个变量来存储Json文件的内容。例如,可以使用类型为“STRING”或“WSTRING”的变量。
5. 使用“FileOpen”函数块打开Json文件,并将其内容读入到变量中。
6. 使用JsonParser函数块中的“ParseJson”函数解析Json文件。此函数将Json文件的内容作为输入,并输出一个Json对象。
7. 使用Json对象中的函数和属性来访问和处理Json数据。
注意:在使用Json库之前,请确保已经安装了最新版本的Codesys。此外,代码中还需要添加一些错误处理程序以确保Json文件正确解析。
相关问题
在Codesys中解析json 文件
在CODESYS中解析JSON文件,可以使用CODESYS提供的JSON库。以下是一个示例程序,演示如何读取一个包含JSON格式数据的文件:
```pascal
VAR
hFile : FILE_HANDLE;
sJsonData : STRING;
hJsonParser : JSON_PARSER_HANDLE;
hJsonNode : JSON_NODE_HANDLE;
sValue : STRING;
nValue : INT;
bValue : BOOL;
END_VAR
hFile := FileOpen('file.json', 'r'); // 打开JSON文件
IF hFile <> 0 THEN // 如果文件打开成功
sJsonData := FileReadString(hFile); // 读取文件内容到字符串变量中
FileClose(hFile); // 关闭文件
hJsonParser := JSON_CreateParser(); // 创建JSON解析器
hJsonNode := JSON_Parse(hJsonParser, ADR(sJsonData)); // 解析JSON数据
IF hJsonNode <> 0 THEN // 如果解析成功
// 读取JSON节点中的数据
sValue := JSON_GetStringValue(JSON_GetNode(hJsonNode, 'name'));
nValue := JSON_GetIntValue(JSON_GetNode(hJsonNode, 'age'));
bValue := JSON_GetBoolValue(JSON_GetNode(hJsonNode, 'isStudent'));
// ...
JSON_DeleteNode(hJsonNode); // 删除JSON节点
END_IF
JSON_DestroyParser(hJsonParser); // 销毁JSON解析器
END_IF
```
在以上示例程序中,`FileOpen`函数用于打开JSON文件,`FileReadString`函数用于读取文件内容到字符串变量中,`JSON_CreateParser`函数用于创建JSON解析器,`JSON_Parse`函数用于解析JSON数据,`JSON_GetNode`函数用于获取JSON节点,`JSON_GetStringValue`、`JSON_GetIntValue`、`JSON_GetBoolValue`等函数用于读取JSON节点中的数据,`JSON_DeleteNode`函数用于删除JSON节点,`JSON_DestroyParser`函数用于销毁JSON解析器。
结合代码描述如何在Codesys中解析json 文件
在CODESYS中解析JSON文件可以使用JSON库,以下是一个示例代码:
```pascal
PROGRAM JSONParsing
VAR
jsonFile : TcFile;
jsonString : STRING;
jsonDecoder : TcJSONDecoder;
jsonObject : TcJSON;
jsonStringValue : TcJSONStringValue;
jsonIntValue : TcJSONIntValue;
jsonArray : TcJSONArray;
i : INT;
BEGIN
// Open the JSON file
jsonFile := SysFileOpenRead('path/to/json/file.json');
IF jsonFile = 0 THEN
// Failed to open file
RETURN;
END_IF
// Read the file into a string
SysFileReadText(jsonFile, jsonString);
// Close the file
SysFileClose(jsonFile);
// Decode the JSON string into a JSON object
jsonDecoder.Decode(jsonString, jsonObject);
// Get a string value from the JSON object
jsonObject.Get('stringKey', jsonStringValue);
IF jsonStringValue <> 0 THEN
// Do something with the string value
// ...
END_IF
// Get an integer value from the JSON object
jsonObject.Get('intKey', jsonIntValue);
IF jsonIntValue <> 0 THEN
// Do something with the integer value
// ...
END_IF
// Get an array from the JSON object
jsonObject.Get('arrayKey', jsonArray);
IF jsonArray <> 0 THEN
FOR i := 0 TO jsonArray.Count - 1 DO
// Do something with each element in the array
// ...
END_FOR
END_IF
END_PROGRAM
```
在上述代码中,使用`SysFileOpenRead`打开JSON文件,使用`SysFileReadText`将文件读取为字符串。然后使用`TcJSONDecoder`将字符串解码为`TcJSON`对象,可以使用`Get`函数获取JSON对象中的字符串、整数或数组。
阅读全文
相关推荐
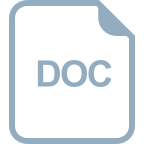
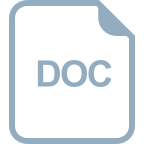
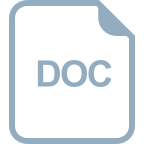





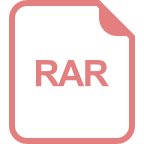
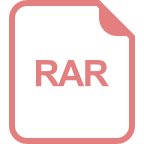
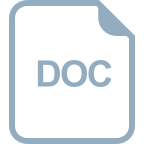
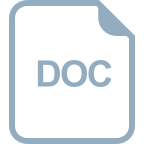
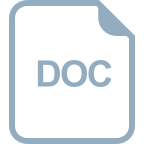
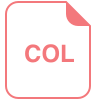


