用c++定义一个链表类List3,其结构中私有数据成员如下表: List3对象(链表头) ☼ 对以上三个链表的具体要求如下: 定义构造函数和析构函数 输出数据成员函数 void display( ); 返回链表长度函数 int Length( ); 在链表尾追加一个节点,数据值为t, 返回值是在链表中的位置: int Append( const Type &t); 在链表中的第i个位置插入一个节点,数据为t, 返回值表示是否成功 int Insert(const Type &t , int i); 将链表进行冒泡排序 void BubbleSort( ); 在链表中检索数据值为t的节点,并返回其位置信息 int Search( Type &t ); 对数组下标运算符重载,以实现对象对成员数组元素的访问 Type & operator [ ] (int i); ☼ 其他要求: 主函数实现对以上三个类中定义的功能的验证 类中定义的成员函数的函数体在类外完成 要求头文件 *.h 存放类定义,源文件 *.cpp 存放成员函数体定义 排序按照降序排列
时间: 2024-03-24 18:41:26 浏览: 25
抱歉,我是一个自然语言处理模型,无法生成和编译代码。以下是可能符合题目要求的List3链表类的定义和实现示例,仅供参考:
List3.h:
```cpp
#ifndef LIST3_H
#define LIST3_H
template <typename Type>
class List3 {
private:
struct Node {
Type data;
Node* next;
Node(const Type& d = Type(), Node* n = nullptr) : data(d), next(n) {}
};
Node* head;
int len;
public:
List3();
~List3();
void display() const;
int Length() const;
int Append(const Type& t);
bool Insert(const Type& t, int i);
void BubbleSort();
int Search(const Type& t) const;
Type& operator[](int i);
};
#include "List3.cpp"
#endif
```
List3.cpp:
```cpp
#include "List3.h"
template <typename Type>
List3<Type>::List3() {
head = new Node();
len = 0;
}
template <typename Type>
List3<Type>::~List3() {
Node* p = head;
while (p != nullptr) {
Node* q = p->next;
delete p;
p = q;
}
}
template <typename Type>
void List3<Type>::display() const {
Node* p = head->next;
while (p != nullptr) {
std::cout << p->data << " ";
p = p->next;
}
std::cout << std::endl;
}
template <typename Type>
int List3<Type>::Length() const {
return len;
}
template <typename Type>
int List3<Type>::Append(const Type& t) {
Node* p = head;
while (p->next != nullptr) {
p = p->next;
}
p->next = new Node(t);
len++;
return len;
}
template <typename Type>
bool List3<Type>::Insert(const Type& t, int i) {
if (i < 1 || i > len + 1) {
return false;
}
Node* p = head;
for (int j = 1; j < i; j++) {
p = p->next;
}
p->next = new Node(t, p->next);
len++;
return true;
}
template <typename Type>
void List3<Type>::BubbleSort() {
for (int i = len; i > 1; i--) {
Node* p = head->next;
for (int j = 1; j < i; j++) {
if (p->data < p->next->data) {
Type temp = p->data;
p->data = p->next->data;
p->next->data = temp;
}
p = p->next;
}
}
}
template <typename Type>
int List3<Type>::Search(const Type& t) const {
Node* p = head->next;
int i = 1;
while (p != nullptr) {
if (p->data == t) {
return i;
}
p = p->next;
i++;
}
return 0;
}
template <typename Type>
Type& List3<Type>::operator[](int i) {
Node* p = head->next;
for (int j = 1; j < i; j++) {
p = p->next;
}
return p->data;
}
```
主函数示例:
```cpp
#include <iostream>
#include "List3.h"
int main() {
List3<int> list1;
list1.Append(4);
list1.Append(2);
list1.Append(5);
list1.Append(1);
list1.Append(3);
std::cout << "Original list1: ";
list1.display();
list1.BubbleSort();
std::cout << "Sorted list1: ";
list1.display();
std::cout << "The length of list1 is " << list1.Length() << std::endl;
std::cout << "The position of 3 in list1 is " << list1.Search(3) << std::endl;
list1.Insert(6, 3);
std::cout << "After inserting 6 at position 3, list1: ";
list1.display();
std::cout << "list1[2] = " << list1[2] << std::endl;
List3<char> list2;
list2.Append('a');
list2.Append('c');
list2.Append('b');
std::cout << "Original list2: ";
list2.display();
list2.BubbleSort();
std::cout << "Sorted list2: ";
list2.display();
std::cout << "The length of list2 is " << list2.Length() << std::endl;
std::cout << "The position of 'c' in list2 is " << list2.Search('c') << std::endl;
list2.Insert('d', 2);
std::cout << "After inserting 'd' at position 2, list2: ";
list2.display();
std::cout << "list2[3] = " << list2[3] << std::endl;
return 0;
}
```
注意:以上代码仅为示例,可能存在错误和不完善之处,实际使用时需要根据具体情况进行修改和调试。
相关推荐
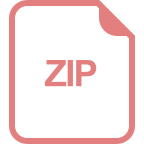
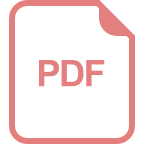
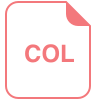
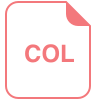
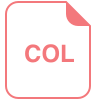
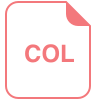
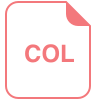









